C#: Create a structure and assign the value and call it
Write a C# Sharp program to create a structure, assign a value to it, and call it.
Sample Solution:-
C# Sharp Code:
using System.IO;
using System;
// Declaration of a class named aNewClass
class aNewClass
{
public int x; // Member variable x
public int y; // Member variable y
}
// Declaration of a structure named aNewStruc
struct aNewStruc
{
public int x; // Member variable x
public int y; // Member variable y
}
class strucExer4
{
static void Main(string[] args)
{
// Displaying a message indicating the creation of a structure, assigning values, and calling it
Console.Write("\n\nCreate a structure and assign the value and call it :\n");
Console.Write("---------------------------------------------------------\n");
// Creating an instance of the aNewClass class and assigning values to its members
aNewClass ClassNum1 = new aNewClass();
ClassNum1.x = 75;
ClassNum1.y = 95;
// Creating another instance of aNewClass and assigning it the value of ClassNum1
aNewClass ClassNum2 = ClassNum1;
// Modifying the values of ClassNum1
ClassNum1.x = 7500;
ClassNum1.y = 9500;
// Displaying values of ClassNum2 after modifying ClassNum1
Console.WriteLine("\nAssign in Class: x:{0}, y:{1}", ClassNum2.x, ClassNum2.y);
// Creating an instance of the aNewStruc structure and assigning values to its members
aNewStruc StrucNum1 = new aNewStruc();
StrucNum1.x = 750;
StrucNum1.y = 950;
// Creating another instance of aNewStruc and assigning it the value of StrucNum1
aNewStruc StrucNum2 = StrucNum1;
// Modifying the values of StrucNum1
StrucNum1.x = 75;
StrucNum1.y = 95;
// Displaying values of StrucNum2 after modifying StrucNum1
Console.WriteLine("Assign in Structure: x:{0}, y:{1}\n\n", StrucNum2.x, StrucNum2.y);
}
}
Sample Output:
Create a structure and assign the value and call it : --------------------------------------------------------- Assign in Class: x:7500, y:9500 Assign in Structure: x:750, y:950
Flowchart:
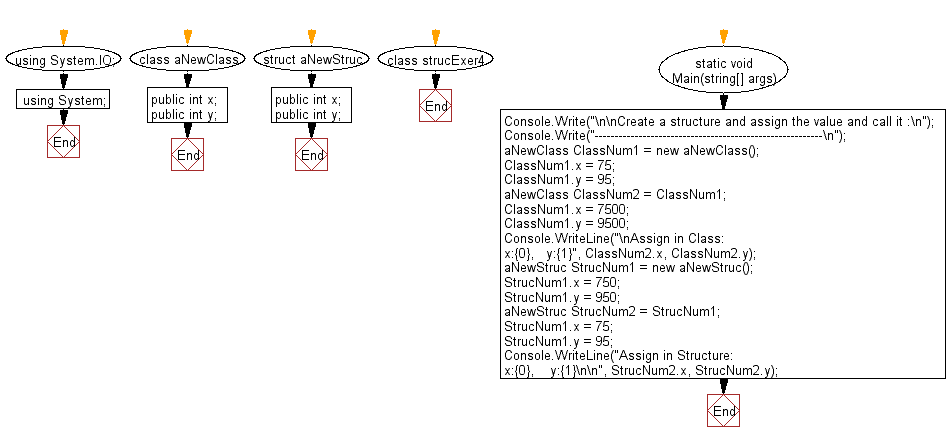
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to create a nested struct to store two data for an employee in an array.
Next: Write a program in C# Sharp to show what happen when a struct and a class instance is passed to a method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.