C#: Structure initialization without using the new operator
Write a program in C# Sharp to demonstrates structure initialization without using the new operator.
Sample Solution:-
C# Sharp Code:
using System;
// Declaration of a public structure named newStruct
public struct newStruct
{
public int m, n; // Public fields 'm' and 'n' within the structure
// Parameterized constructor for the newStruct
public newStruct(int pt1, int pt2)
{
m = pt1; // Initializing 'm' with the value of pt1
n = pt2; // Initializing 'n' with the value of pt2
}
}
// Main class
class strucExer8
{
// Main method
static void Main()
{
// Displaying a message indicating structure initialization without using the new operator
Console.Write("\n\nStructure initialization without using the new operator :\n");
Console.Write("----------------------------------------------------------\n");
// Declaring an instance of newStruct without using the new operator
newStruct myPoint;
// Assigning values directly to the fields 'm' and 'n'
myPoint.m = 30;
myPoint.n = 40;
// Displaying the values of fields m and n for myPoint instance
Console.Write("\nnewStruct : ");
Console.WriteLine("m = {0}, n = {1}", myPoint.m, myPoint.n);
Console.WriteLine("\nPress any key to exit.");
Console.ReadKey();
}
}
Sample Output:
Structure initialization without using the new operator : ---------------------------------------------------------- newStruct : m = 30, n = 40 Press any key to exit.
Flowchart:
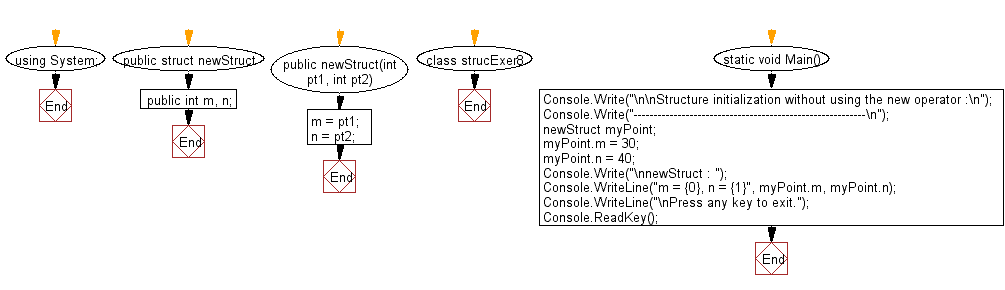
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to demonstrates structure initialization using both default and parameterized constructors.
Next: Write a program in C# Sharp to insert the information of two books.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.