Java: Arrange the elements of a given array of integers where all positive integers appear before all the negative integers
Java Array: Exercise-49 with Solution
Write a Java program to arrange the elements of an array of integers so that all positive integers appear before all negative integers.
Pictorial Presentation:
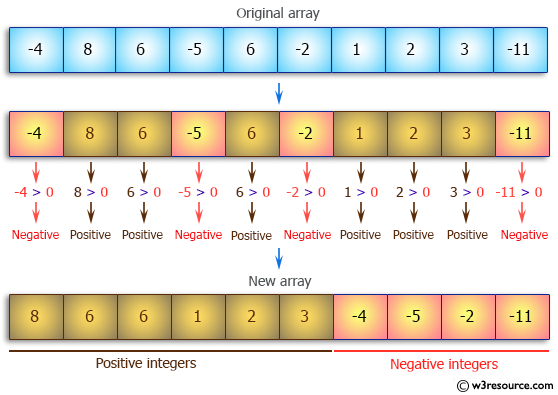
Sample Solution:
Java Code:
// Import the necessary Java utility class for working with arrays.
import java.util.Arrays;
// Define the Main class.
public class Main {
// The main method for executing the program.
public static void main(String[] args) {
// Define an array of integers.
int arra_nums[] = {-4, 8, 6, -5, 6, -2, 1, 2, 3, -11};
// Print the original array.
System.out.println("Original array: " + Arrays.toString(arra_nums));
int j, temp, arr_size;
// Get the size of the array.
arr_size = arra_nums.length;
for (int i = 0; i < arr_size; i++) {
j = i;
// Shift positive numbers to the left and negative numbers to the right.
while ((j > 0) && (arra_nums[j] > 0) && (arra_nums[j - 1] < 0)) {
temp = arra_nums[j];
arra_nums[j] = arra_nums[j - 1];
arra_nums[j - 1] = temp;
j--;
}
}
// Print the modified array.
System.out.println("New array: " + Arrays.toString(arra_nums));
}
}
Sample Output:
Original array : [-4, 8, 6, -5, 6, -2, 1, 2, 3, -11] New array : [8, 6, 6, 1, 2, 3, -4, -5, -2, -11]
Flowchart:
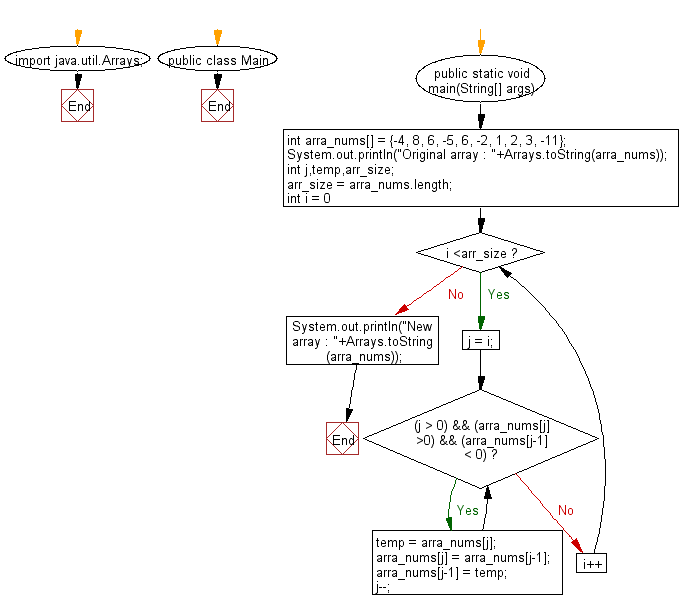
Java Code Editor:
Previous: Write a Java program to arrange the elements of a given array of integers where all negative integers appear before all the positive integers.
Next: Write a Java program to sort an array of positive integers of a given array, in the sorted array the value of the first element should be maximum, second value should be minimum value, third should be second maximum, fourth second be second minimum and so on.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics