Java: Check if a group of numbers at the start and end of a given array are same
Java Basic: Exercise-105 with Solution
Write a Java program to check if a group of numbers (l) at the start and end of a given array are the same.
Pictorial Presentation:
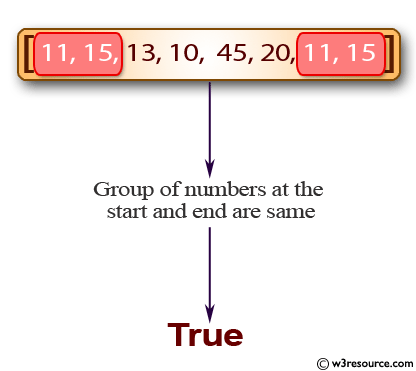
Sample Solution:
Java Code:
import java.util.*;
public class Exercise105 {
public static void main(String[] args) {
int[] array_nums = {11, 15, 13, 10, 45, 20, 11, 15};
System.out.println("Original Array: " + Arrays.toString(array_nums));
int result = 0;
int l = 2;
int start = 0;
int end = array_nums.length - l;
// Check if the elements at corresponding positions within the range [start, end] are equal.
for (; l > 0; l--) {
if (array_nums[start] != array_nums[end]) {
result = 1;
} else {
start++;
end++;
}
}
if (result == 1) {
System.out.printf(String.valueOf(false));
} else {
System.out.printf(String.valueOf(true));
}
System.out.printf("\n");
}
}
Sample Output:
Original Array: [11, 15, 13, 10, 45, 20, 11, 15] true
Flowchart:
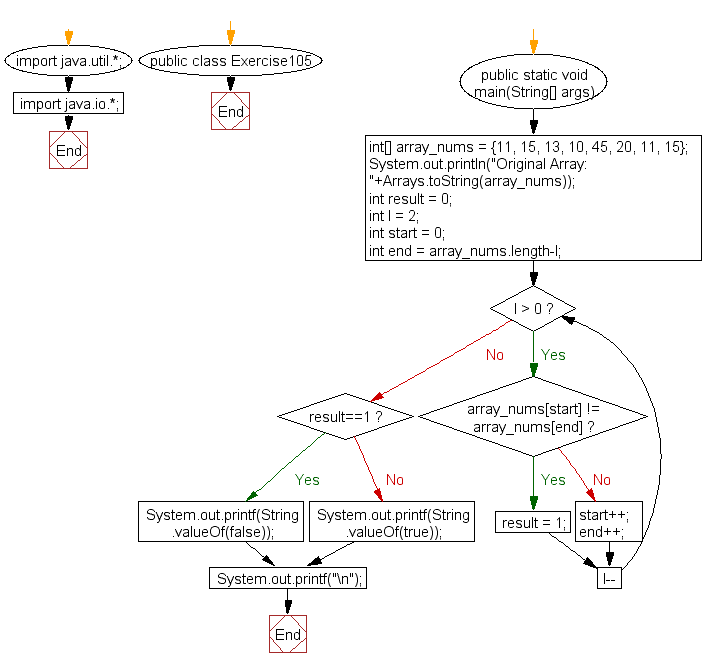
Java Code Editor:
Previous: Write a Java program to create a new array from a given array of integers, new array will contain the elements from the given array before the last element value 10.
Next: Write a Java program to create a new array that is left shifted from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics