Java: Compute the number of trailing zeros in a factorial
Java Basic: Exercise-112 with Solution
Trailing Zeros in Factorial
Write a Java program to compute the number of trailing zeros in a factorial.
Example
7! = 5040, therefore the output should be 1.
Pictorial Presentation:
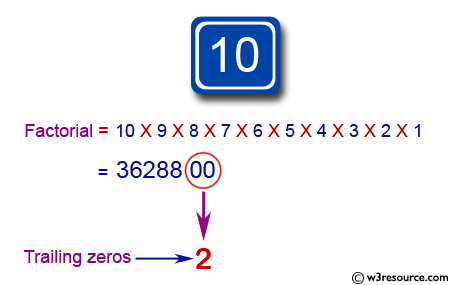
Sample Solution:
Java Code:
import java.util.Scanner;
public class Example112 {
public static void main(String[] arg) {
Scanner in = new Scanner(System.in); // Create a Scanner object for user input
System.out.print("Input a number: "); // Prompt the user to input a number
int n = in.nextInt(); // Read and store the user's input as 'n'
int n1 = n; // Create a copy of 'n' to preserve the original value
long ctr = 0; // Initialize a variable 'ctr' to count trailing zeros
while (n != 0) {
ctr += n / 5; // Count the number of trailing zeros by dividing 'n' by 5 and accumulating the result
n /= 5; // Reduce 'n' by dividing it by 5 for the next iteration
}
System.out.printf("Number of trailing zeros of the factorial %d is %d ", n1, ctr); // Print the result
System.out.printf("\n"); // Print a new line
}
}
Sample Output:
Input a number : 5040 Number of trailing zeros of the factorial 5040 is 1258
Flowchart:
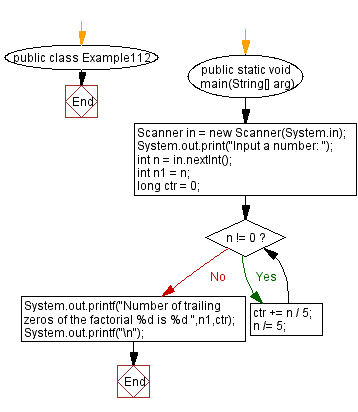
Java Code Editor:
Previous: Write a Java program to add two numbers without using any arithmetic operators.
Next: Write a Java program to merge two given sorted array of integers and create a new sorted array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-112.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics