Java: Check if a positive number is a palindrome or not
Java Basic: Exercise-115 with Solution
Check Palindrome Number
Write a Java program to check if a positive number is a palindrome or not.
Pictorial Presentation:
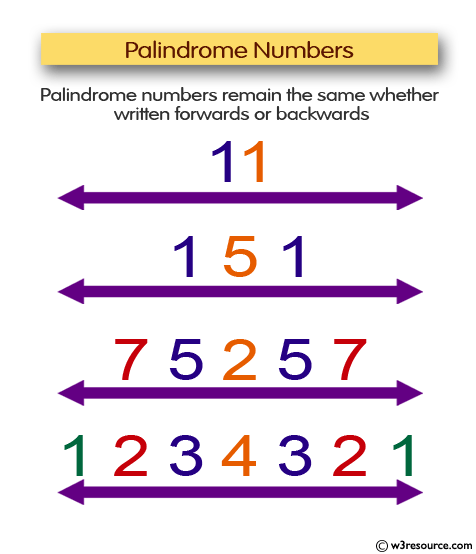
Sample Solution:
Java Code:
import java.util.*;
public class test {
public static void main(String[] args) {
int num;
// Create a Scanner object for user input
Scanner in = new Scanner(System.in);
// Prompt the user for a positive integer
System.out.print("Input a positive integer: ");
// Read the integer entered by the user
int n = in.nextInt();
// Display a message to check if the number is a palindrome
System.out.printf("Is %d a palindrome number?\n", n);
// Check if the number is a palindrome and print the result
System.out.println(is_Palindrome(n));
}
// Function to reverse the digits of a number
public static int reverse_nums(int n) {
int reverse = 0;
while (n != 0) {
reverse *= 10;
reverse += n % 10;
n /= 10;
}
return reverse;
}
// Function to check if a number is a palindrome
public static boolean is_Palindrome(int n) {
return (n == reverse_nums(n));
}
}
Sample Output:
Input a positive integer: 151 Is 151 is a palindrome number? true
Flowchart:
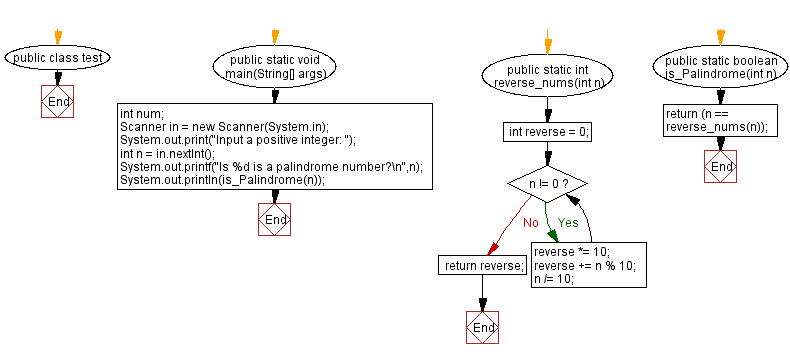
Java Code Editor:
Previous: Write a Java program to given a string and an offset, rotate string by offset (rotate from left to right).
Next: Write a Java program which iterates the integers from 1 to 100. For multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". When number is divided by both three and five, print "fizz buzz".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-115.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics