Java: Compute the square root of a given integer
Java Basic: Exercise-117 with Solution
Square Root Calculation
Write a Java program to compute the square root of a given number.
Pictorial Presentation:
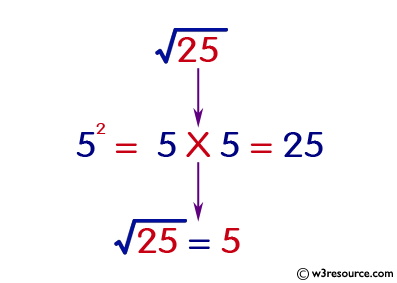
Sample Solution:
Java Code:
import java.util.*;
public class Exercise117 {
public static void main(String[] args) {
int num;
Scanner in = new Scanner(System.in);
// Prompt the user to input a positive integer
System.out.print("Input a positive integer: ");
int n = in.nextInt();
// Print a message indicating the calculation about to take place
System.out.printf("Square root of %d is: ", n);
// Call the sqrt method to calculate the square root and print the result
System.out.println(sqrt(n));
}
// Method to calculate the square root of a number
private static int sqrt(int num) {
if (num == 0 || num == 1) {
return num;
}
int a = 0;
int b = num;
// Perform a binary search to find the square root
while (a <= b) {
int mid = (a + b) >> 1;
if (num / mid < mid) {
b = mid - 1;
} else {
if (num / (mid + 1) <= mid) {
return mid;
}
a = mid + 1;
}
}
return a;
}
}
Sample Output:
Input a positive integer: 25 Square root of 25 is: 5
Flowchart:
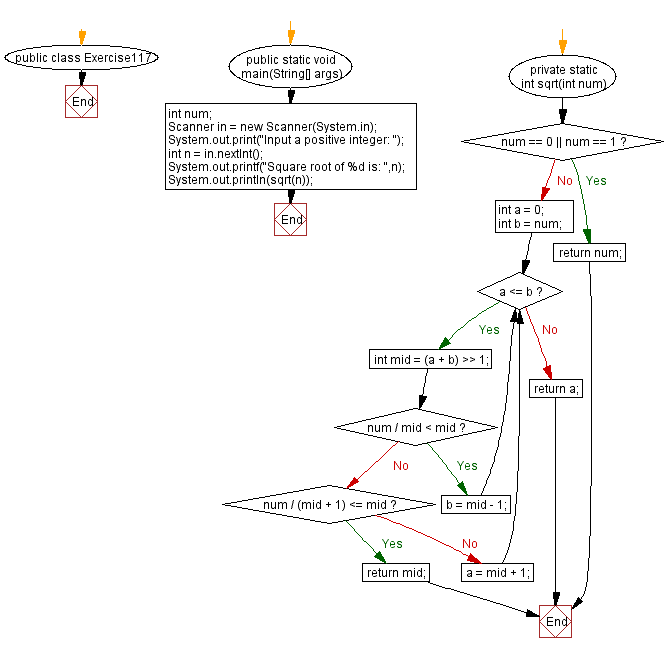
Java Code Editor:
Previous: Write a Java program which iterates the integers from 1 to 100. For multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". When number is divided by both three and five, print "fizz buzz".
Next: Write a Java program to get the first occurrence (Position starts from 0.) of a string within a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-117.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics