Java: Calculate the median of a given unsorted array of integers
Java Basic: Exercise-128 with Solution
Median of Array
Write a Java program to calculate the median of a non-sorted array of integers.
Example: {10,2,38,23,38,23,21}
Output: 23
Pictorial Presentation:
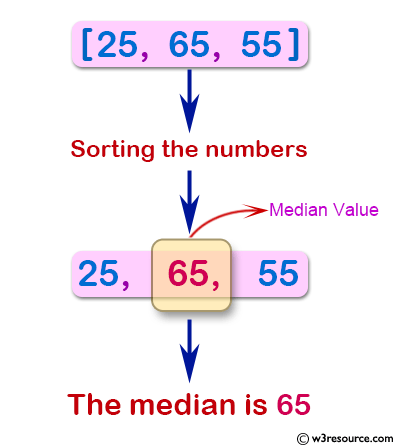
Sample Solution:
Java Code:
import java.util.*;
public class Main {
public static void main(String[] args) {
// Define and initialize an array of integers
int[] nums = {10, 2, 38, 22, 38, 23};
// Display the original array
System.out.println("Original array: " + Arrays.toString(nums));
// Calculate and display the median of the array
System.out.println("Median of the said array of integers: " + getMedian(nums));
// Define and initialize another array of integers
int[] nums1 = {10, 2, 38, 23, 38, 23, 21};
// Display the original array
System.out.println("\nOriginal array: " + Arrays.toString(nums1));
// Calculate and display the median of the second array
System.out.println("Median of the said array of integers: " + getMedian(nums1));
}
public static int getMedian(int[] array) {
// Check if the length of the array is even
if (array.length % 2 == 0) {
// Calculate the median for even-sized arrays
int mid = array.length / 2;
return (array[mid] + array[mid - 1]) / 2;
}
// Calculate the median for odd-sized arrays
return array[array.length / 2];
}
}
Sample Output:
Original array: [10, 2, 38, 22, 38, 23] Median of the said array of integers: 30 Original array: [10, 2, 38, 23, 38, 23, 21] Median of the said array of integers: 23
Flowchart:
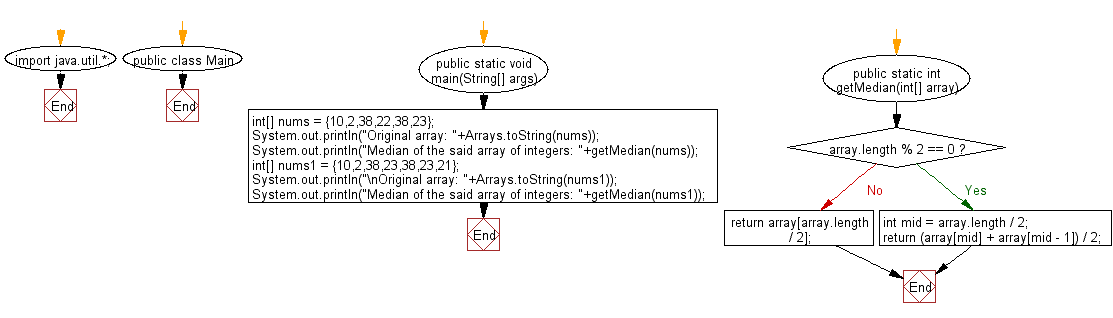
Java Code Editor:
Previous: Write a Java program to get the Postorder traversal of its nodes' values of a given a binary tree.
Next: Write a Java program to find a number that appears only once in a given array of integers, all numbers occur twice.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-128.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics