Java: Find the maximum sum of a contiguous subsequence from a given sequence of numbers
Java Basic: Exercise-223 with Solution
Maximum Sum of Contiguous Subsequence
Write a Java program to find the maximum sum of a contiguous subsequence from a given sequence of numbers a1, a2, a3, ... an. A subsequence of one element is also a continuous subsequence.
Input:
You can assume that 1 ≤ n ≤ 5000 and -100000 ≤ ai ≤ 100000.
Input numbers are separated by a space.
Input 0 to exit.
Visual Presentation:
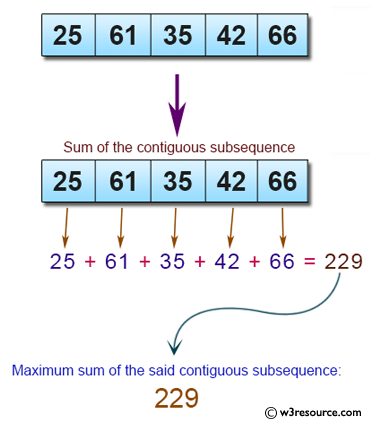
Sample Solution:
Java Code:
// Importing necessary Java utilities for input
import java.util.*;
// Main class named "Main"
public class Main {
// Main method to execute the program
public static void main(String [] args) {
// Creating a Scanner object for user input
Scanner s = new Scanner(System.in);
// Prompting the user to specify the number of integers to input
System.out.println("How many integers would you like to input?");
int n = s.nextInt();
// Initializing variables for the maximum sum and the current accumulation
int ans = -100000;
int acc = 0;
// Prompting the user to input the integers
System.out.println("Input the integers:");
// Looping through each input integer to find the maximum contiguous subsequence sum
for (int i = 0; i < n; i++) {
// Accumulating the current integer
acc += s.nextInt();
// Updating the maximum sum using Math.max function
ans = Math.max(ans, acc);
// Resetting the accumulation to 0 if it becomes negative
if (acc < 0) acc = 0;
}
// Outputting the maximum sum of the contiguous subsequence
System.out.println("Maximum sum of the said contiguous subsequence:");
System.out.println(ans);
}
}
Sample Output:
How many integers would you like to input? 5 Input the integers: 25 61 35 42 66 Maximum sum of the said contiguous subsequence: 229
Flowchart:
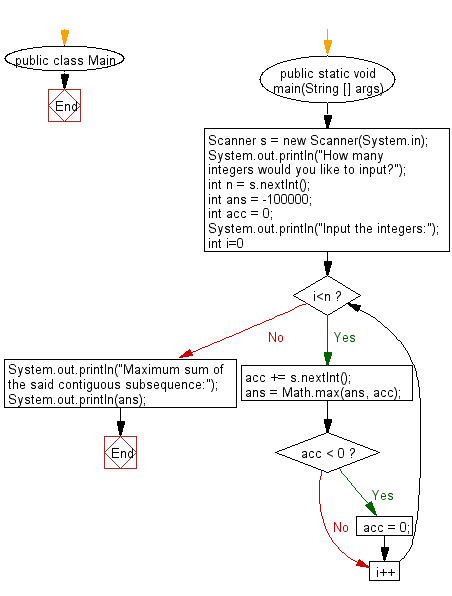
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to test whether two lines PQ and RS are parallel. The four points are P(x1, y1), Q(x2, y2), R(x3, y3), S(x4, y4).
Next: There are two circles C1 with radius r1, central coordinate (x1, y1) and C2 with radius r2 and central coordinate (x2, y2)
Write a Java program to test the followings -
"C2 is in C1" if C2 is in C1
"C1 is in C2" if C1 is in C2
"Circumference of C1 and C2 intersect" if circumference of C1 and C2 intersect, and
"C1 and C2 do not overlap" if C1 and C2 do not overlap.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-223.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics