Java: Accepts a string from the user and check whether the string is correct or not
Java Basic: Exercise-244 with Solution
Write a Java program that accepts a string from the user and checks whether it is correct or not.
The conditions for getting the "correct answer" are:
a) There must be only three characters X, Y, and Z in the string, and no other characters.
b) Any string of any form such as aXYZa can get the "correct answer", where a is either an empty string or a string consisting only of the letter X;
c) If aXbZc is true, aXbYZca is also valid, where a, b, c are either empty strings or a string consisting only of the letter X.
Visual Presentation:
Sample Solution:
Java Code:
// Importing necessary classes
import java.util.PriorityQueue;
import java.util.Scanner;
// Defining a class named "Main"
public class Main {
// Static nested class "Dic" representing a pair of word and page number
static class Dic implements Comparable{
// Instance variables to store word and page number
String moji;
int page;
// Parameterized constructor to initialize word and page number
Dic(String moji, int page){
this.moji=moji;
this.page=page;
}
// Implementation of the compareTo method to define the natural order of Dic objects
public int compareTo(Dic d) {
if(this.moji.equals(d.moji)) {
return this.page-d.page;
}
else {
return this.moji.compareTo(d.moji);
}
}
}
// Main method, the entry point of the program
public static void main(String[] args) {
// Using the try-with-resources statement to automatically close the Scanner
try(Scanner sc = new Scanner(System.in)){
// Creating a PriorityQueue to store Dic objects
PriorityQueue pq=new PriorityQueue<>();
// Prompting the user to input pairs of a word and a page number
System.out.println("Input pairs of a word and a page number:");
// Reading input until there is no more input
while(sc.hasNextLine()) {
// Reading a line and splitting it into word and page number
String str=sc.nextLine();
String[] token=str.split(" ");
String s=token[0];
int n=Integer.parseInt(token[1]);
// Creating a new Dic object and adding it to the PriorityQueue
pq.add(new Dic(s, n));
}
// Initializing a variable to store the previous word
String pre="";
// Printing the word and page number in alphabetical order
System.out.println("\nWord and page number in alphabetical order:");
while(!pq.isEmpty()) {
// Polling the smallest Dic object from the PriorityQueue
Dic dic=pq.poll();
// Checking if the current word is the same as the previous one
if(dic.moji.equals(pre)) {
System.out.print(" "+dic.page);
}
else if(pre.equals("")) {
System.out.println(dic.moji);
System.out.print(dic.page);
}
else {
System.out.println();
System.out.println(dic.moji);
System.out.print(dic.page);
}
// Updating the previous word
pre=dic.moji;
}
System.out.println();
}
}
}
Sample Output:
Input a string: XYZ Correct format..
Flowchart:
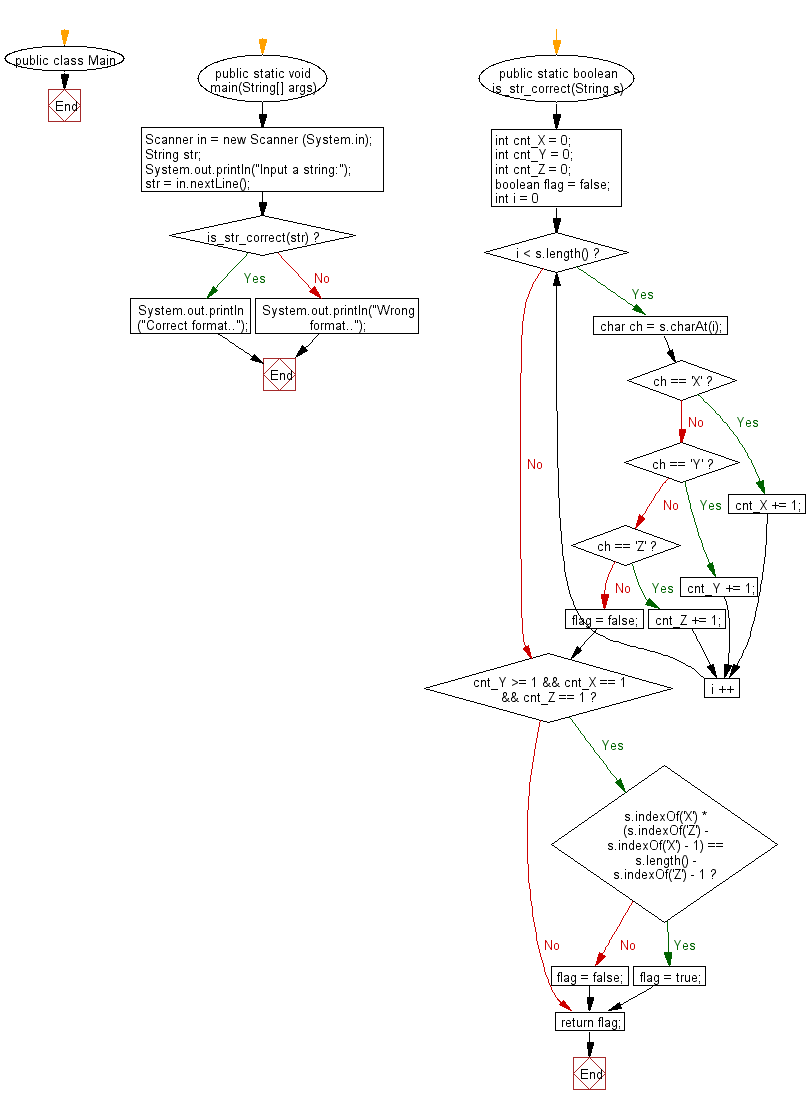
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
Next: Write a Java program which accepts students name, id, and marks and display the highest score and the lowest score.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics