Java: Display the product of two numbers
Java Basic: Exercise-5 with Solution
Write a Java program that takes two numbers as input and displays the product of two numbers.
Test Data:
Input first number: 25
Input second number: 5
Pictorial Presentation:
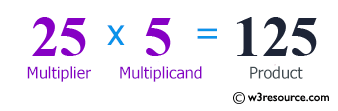
Sample Solution-1
Java Code:
import java.util.Scanner;
public class Exercise5 {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner in = new Scanner(System.in);
// Prompt the user to input the first number
System.out.print("Input first number: ");
// Read and store the first number
int num1 = in.nextInt();
// Prompt the user to input the second number
System.out.print("Input second number: ");
// Read and store the second number
// Calculate the product of the two numbers and display the result
System.out.println(num1 + " x " + num2 + " = " + num1 * num2);
}
}
Explanation:
The above Java code takes two integer numbers as input from the user, multiplies them, and then displays the result in the format "num1 x num2 = result." It uses the "Scanner" class to read user input and performs multiplication on the input values.
Sample Output:
Input first number: 25 Input second number: 5 25 x 5 = 125
Flowchart:
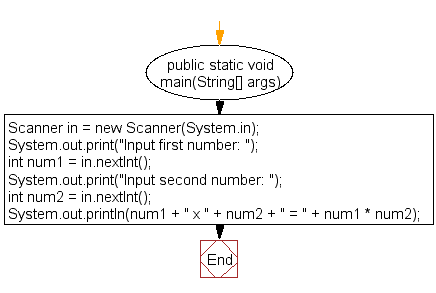
Sample Solution-2
Java Code:
public class Main
{
// Declare and initialize static variables x and y
static int x = 25;
static int y = 5;
public static void main(String[] args)
{
// Calculate and print the product of x and y
System.out.println(x * y);
}
}
Sample Output:
125
Flowchart:
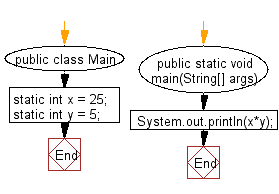
Java Code Editor:
Previous: Write a Java program to print the result of the specified operations.
Next: Write a Java program to print the sum (addition), multiply, subtract, divide and remainder of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics