Java: Compare two hash set
Write a Java program to compare two hash sets.
Sample Solution:
Java Code:
import java.util.*;
public class Exercise10 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
HashSet<String>h_set2 = new HashSet<String>();
h_set2.add("Red");
h_set2.add("Pink");
h_set2.add("Black");
h_set2.add("Orange");
//comparison output in hash set
HashSet<String>result_set = new HashSet<String>();
for (String element : h_set){
System.out.println(h_set2.contains(element) ? "Yes" : "No");
}
}
}
Sample Output:
Yes No Yes No
Pictorial Presentation:
Flowchart:
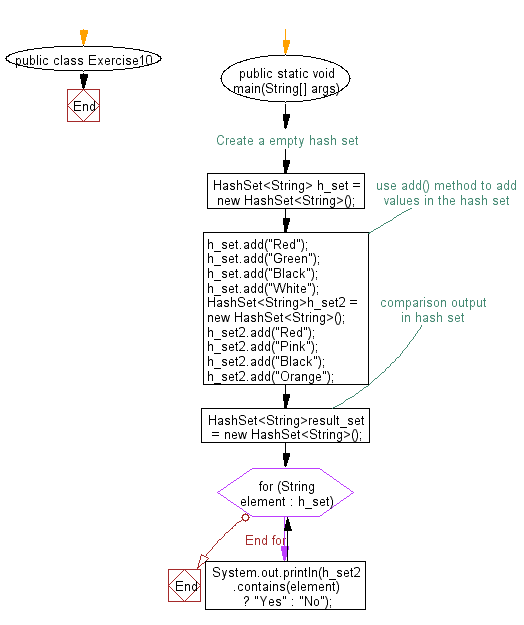
For more Practice: Solve these Related Problems:
- Write a Java program to compare two HashSets for equality using the equals() method.
- Write a Java program to find the differences between two HashSets and print the unique elements of each.
- Write a Java program to compute the intersection of two HashSets using retainAll() and then print the result.
- Write a Java program to compare two HashSets by converting them to sorted arrays and then comparing element by element.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Convert a hash set to a List/ArrayList.
Next: Compare two sets and retain elements which are same on both sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.