Java: Iterate through all elements in a hash list
2. Iterate HashSet Elements
Write a Java program to iterate through all elements in a hash list.
Sample Solution:
Java Code:
import java.util.*;
import java.util.Iterator;
public class Exercise2 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
h_set.add("Pink");
h_set.add("Yellow");
// set Iterator
Iterator<String> p = h_set.iterator();
// Iterate the hash set
while (p.hasNext()) {
System.out.println(p.next());
}
}
}
Sample Output:
Red White Pink Yellow Black Green
Flowchart:
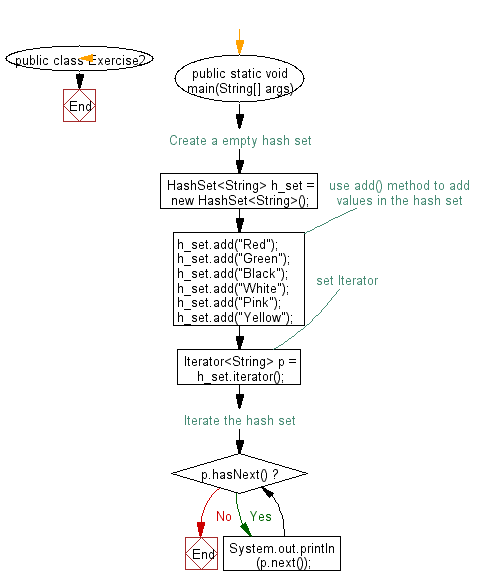
For more Practice: Solve these Related Problems:
- Write a Java program to iterate over a HashSet using an Iterator and print only the elements that satisfy a given condition.
- Write a Java program to iterate through a HashSet using Java 8 forEach() and a lambda expression, printing each element with its hash code.
- Write a Java program to convert a HashSet to a stream, filter the elements by a specific pattern, and then print the filtered results.
- Write a Java program to iterate over a HashSet, convert each element to uppercase, and collect the results into a new HashSet.
Go to:
PREV : Append Element to HashSet.
NEXT : Get HashSet Size.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.