Java: Iterate through all elements in a linked list
Java Collection, LinkedList Exercises: Exercise-2 with Solution
Write a Java program to iterate through all elements in a linked list.
Sample Solution:-
Java Code:
import java.util.LinkedList;
public class Exercise2 {
public static void main(String[] args) {
// create an empty linked list
LinkedList<String> l_list = new LinkedList<String>();
// use add() method to add values in the linked list
l_list.add("Red");
l_list.add("Green");
l_list.add("Black");
l_list.add("White");
l_list.add("Pink");
// Print the linked list
for (String element : l_list) {
System.out.println(element);
}
}
}
Sample Output:
Red Green Black White Pink
Flowchart:
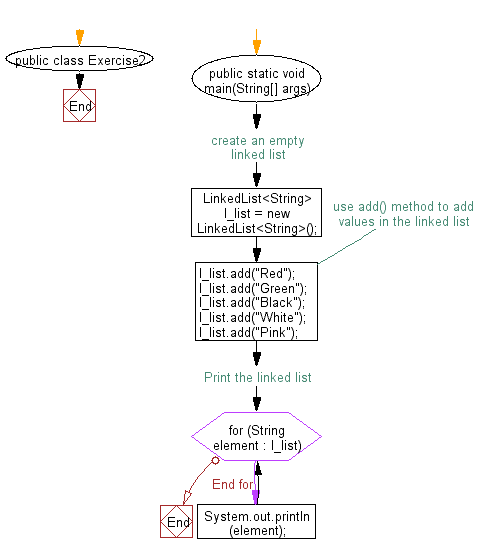
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Append the specified element to the end of a linked list.
Next: Iterate through all elements in a linked list starting at the specified position.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/collection/java-collection-linked-list-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics