Java: Test an linked list is empty or not
Write a Java program to check if a linked list is empty or not.
Sample Solution:-
Java Code:
import java.util.LinkedList;
import java.util.Collections;
public class Exercise25 {
public static void main(String[] args) {
LinkedList<String> c1= new LinkedList<String>();
c1.add("Red");
c1.add("Green");
c1.add("Black");
c1.add("White");
c1.add("Pink");
System.out.println("Original linked list: " + c1);
System.out.println("Check the above linked list is empty or not! "+c1.isEmpty());
c1.removeAll(c1);
System.out.println("Linked list after remove all elements "+c1);
System.out.println("Check the above linked list is empty or not! "+c1.isEmpty());
}
}
Sample Output:
Original linked list: [Red, Green, Black, White, Pink] Check the above linked list is empty or not! false Linked list after remove all elements [] Check the above linked list is empty or not! true
Pictorial Presentation:
Flowchart:
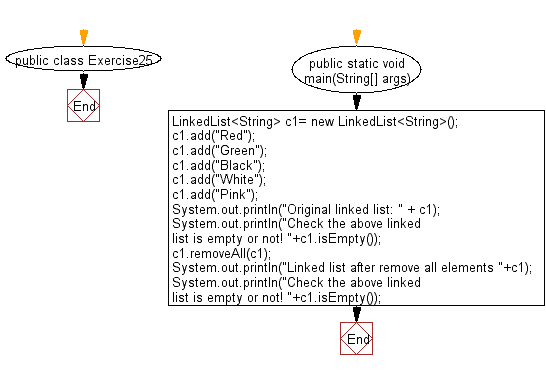
For more Practice: Solve these Related Problems:
- Write a Java program to determine if a linked list is empty using the isEmpty() method and print an appropriate message.
- Write a Java program to use a lambda expression that returns true if a linked list contains no elements.
- Write a Java program to compare two linked lists and check if one is empty while the other is not.
- Write a Java program to implement a recursive method to check if a linked list is empty without using built-in methods.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Compare two linked lists.
Next: Replace an element in a linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.