Java: Insert the specified element at the specified position in the linked list
5. Insert Element at Position
Write a Java program to insert the specified element at the specified position in the linked list.
Sample Solution:-
Java Code:
import java.util.LinkedList;
public class Exercise5 {
public static void main(String[] args) {
// create an empty linked list
LinkedList <String> l_list = new LinkedList <String> ();
// use add() method to add values in the linked list
l_list.add("Red");
l_list.add("Green");
l_list.add("Black");
l_list.add("White");
l_list.add("Pink");
System.out.println("Original linked list: ");
System.out.println("Let add the Yellow color after the Red Color: " + l_list);
l_list.add(1, "Yellow");
// print the list
System.out.println("The linked list:" + l_list);
}
}
Sample Output:
Original linked list: Let add the Yellow color after the Red Color: [Red, Green, Black, White , Pink] The linked list:[Red, Yellow, Green, Black, White, Pink]
Pictorial Presentation:
Flowchart:
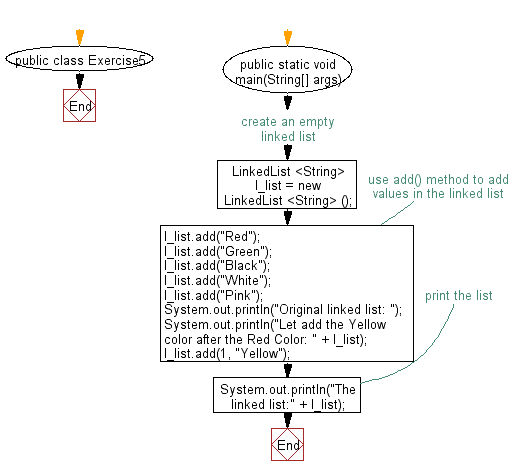
For more Practice: Solve these Related Problems:
- Write a Java program to insert an element at a user-specified index in a linked list and then print the updated list.
- Write a Java program to implement insertion at a specific position using recursion and validate the new position of the element.
- Write a Java program to insert an element at the middle of a linked list and then display the list before and after insertion.
- Write a Java program to insert multiple elements at a given position in a linked list and then verify the ordering.
Go to:
PREV : Iterate in Reverse Order.
NEXT : Insert at First and Last.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.