Java: Get the number of elements in a tree set
Java Collection, TreeSet Exercises: Exercise-7 with Solution
Write a Java program to get the number of elements in a tree set.
Sample Solution:
Java Code:
import java.util.TreeSet;
import java.util.Iterator;
public class Exercise7 {
public static void main(String[] args) {
// create an empty tree set
TreeSet<String> t_set = new TreeSet<String>();
// use add() method to add values in the tree set
t_set.add("Red");
t_set.add("Green");
t_set.add("Black");
t_set.add("Pink");
t_set.add("orange");
System.out.println("Original tree set: " + t_set);
System.out.println("Size of the tree set: " + t_set.size());
}
}
Sample Output:
Original tree set: [Black, Green, Pink, Red, orange] Size of the tree set: 5
Flowchart:
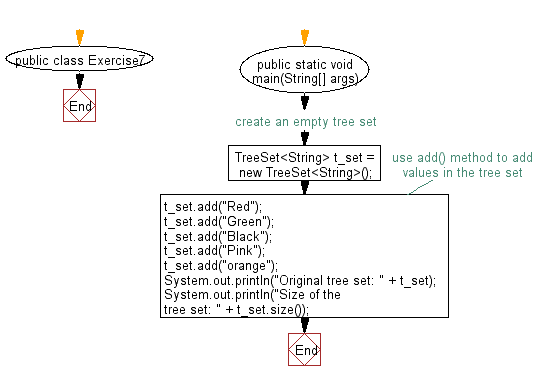
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Clone a tree set list to another tree set.
Next: Compare two tree sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics