Java: Compare two given signed and unsigned numbers
Compare Signed and Unsigned Numbers
Write a Java program to compare two signed and unsigned numbers.
Sample Solution:
Java Code:
public class Main {
public static void main(String[] args) {
int in1 = Integer.MIN_VALUE;
int in2 = Integer.MAX_VALUE;
System.out.println("Signed integers: " + in1 + ", " + in2);
System.out.println("-----------------------------------------");
int compare_Signed_num = Integer.compare(in1, in2);
System.out.println("Result of comparing signed numbers: " + compare_Signed_num);
int compare_Unsigned_num = Integer.compareUnsigned(in1, in2);
System.out.println("Result of comparing unsigned numbers: " + compare_Unsigned_num);
}
}
Sample Output:
Signed integers: -2147483648, 2147483647 ----------------------------------------- Result of comparing signed numbers: -1 Result of comparing unsigned numbers: 1
Flowchart:
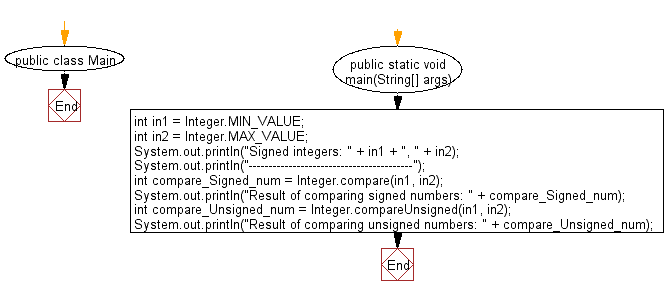
For more Practice: Solve these Related Problems:
- Write a Java program to compare two integers as signed and as unsigned using Java’s Integer methods.
- Write a Java program to sort an array of integers based on their unsigned values.
- Write a Java program to demonstrate the difference between signed and unsigned comparisons by converting negative numbers to their unsigned equivalents.
- Write a Java program to compare two numbers and output the comparison result in both signed and unsigned contexts.
Go to:
PREV : Check Finite Floating-Point Value.
NEXT : Compute Floor Division and Modulus.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.