Java: Print yyyy-MM-dd, HH:mm:ss, yyyy-MM-dd HH:mm:ss, E MMM yyyy HH:mm:ss.SSSZ and HH:mm:ss,Z
Java DateTime, Calendar: Exercise-45 with Solution
Write a Java program to print yyyy-MM-dd, HH:mm:ss, yyyy-MM-dd HH:mm:ss, E MMM yyyy HH:mm:ss.SSSZ and HH:mm:ss,Z format pattern for date and time.
Sample Solution:
Java Code:
import java.text.SimpleDateFormat;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.ZonedDateTime;
import java.time.OffsetTime;
import java.time.format.DateTimeFormatter;
import java.util.Date;
public class Main {
public static void main(String[] args) {
String result;
//yyyy-MM-dd
LocalDate localDate = LocalDate.now();
DateTimeFormatter formatterLocalDate = DateTimeFormatter.ofPattern("yyyy-MM-dd");
result = formatterLocalDate.format(localDate);
System.out.println("\nyyyy-MM-dd: " + result);
// HH:mm:ss
LocalTime localTime = LocalTime.now();
DateTimeFormatter formatterLocalTime = DateTimeFormatter.ofPattern("HH:mm:ss");
result = formatterLocalTime.format(localTime);
System.out.println("\nHH:mm:ss: " + result);
// yyyy-MM-dd HH:mm:ss
LocalDateTime localDateTime = LocalDateTime.now();
DateTimeFormatter formatterLocalDateTime =
DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
result = formatterLocalDateTime.format(localDateTime);
System.out.println("\nyyyy-MM-dd HH:mm:ss: " + result);
// E MMM yyyy HH:mm:ss.SSSZ
ZonedDateTime zonedDateTime = ZonedDateTime.now();
DateTimeFormatter formatterZonedDateTime =
DateTimeFormatter.ofPattern("E MMM yyyy HH:mm:ss.SSSZ");
result = formatterZonedDateTime.format(zonedDateTime);
System.out.println("\nE MMM yyyy HH:mm:ss.SSSZ: " + result);
// HH:mm:ss,Z
OffsetTime offsetTime = OffsetTime.now();
DateTimeFormatter formatterOffsetTime =
DateTimeFormatter.ofPattern("HH:mm:ss,Z");
result = formatterOffsetTime.format(offsetTime);
System.out.println("\nHH:mm:ss,Z: " + result);
}
}
Sample Output:
yyyy-MM-dd: 2021-12-29 HH:mm:ss: 11:02:12 yyyy-MM-dd HH:mm:ss: 2021-12-29 11:02:12 E MMM yyyy HH:mm:ss.SSSZ: Wed Dec 2021 11:02:12.442+0000 HH:mm:ss,Z: 11:02:12,+0000
Flowchart:
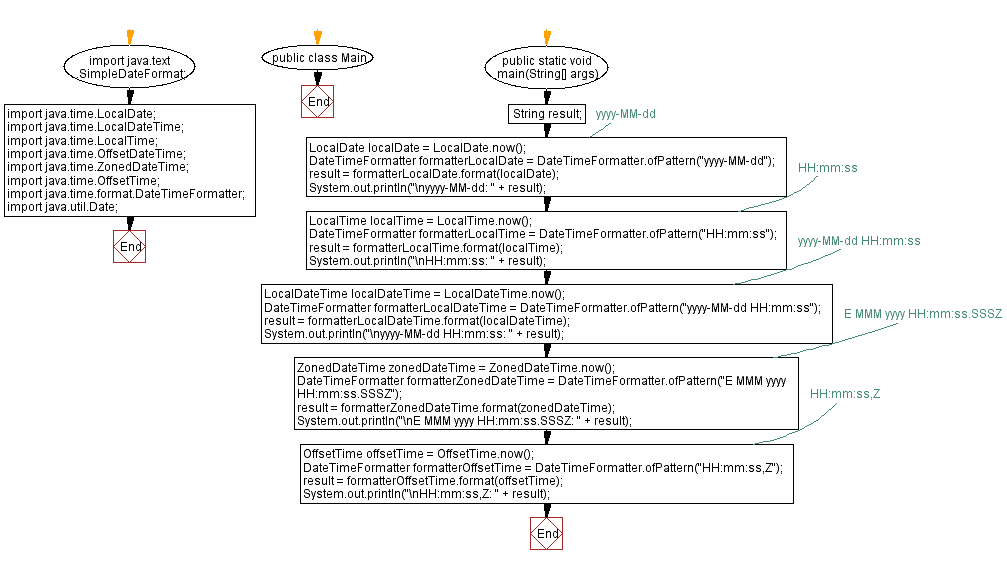
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to define and extract zone offsets.
Next: Write a Java program to count the number of days between two given years.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/datetime/java-datetime-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics