Java Inheritance Programming - Animal with a method called makeSound
Java Inheritance: Exercise-1 with Solution
Write a Java program to create a class called Animal with a method called makeSound(). Create a subclass called Cat that overrides the makeSound() method to bark.
This exercise shows how inheritance works in Java programming language. Inheritance allows you to create new classes based on existing classes, inheriting their attributes and behaviors. In this case, the 'Cat' class is a more specific implementation of the 'Animal' class, adding quarrel behavior.
Sample Solution:
Java Code:
// Animal.java
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound.");
}
}
// Cat.java
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("The cat quarrels.");
}
}
// Main.java
public class Main {
public static void main(String[] args) {
Animal animal = new Animal();
Cat cat = new Cat();
animal.makeSound();
cat.makeSound();
}
}
Sample Output:
The animal makes a sound. The cat quarrels.
Explanation:
The above exercise demonstrates Java programming inheritance. In this program, we create a base class called 'Animal' with a method named makeSound(). Then, you will create a subclass of 'Animal' called 'Cat' which inherits from 'Animal'. The 'Cat' class will override the makeSound() method and change it to make a quarrel sound.
Flowchart:
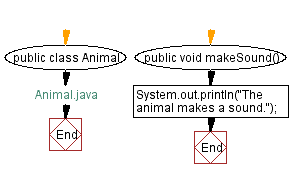
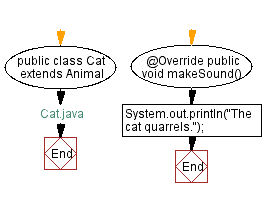
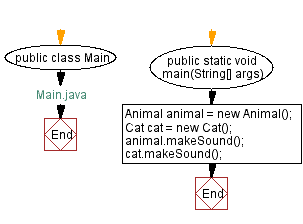
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Java Inheritance Exercises Home.
Next: Create a class called Vehicle with a method called drive(). Create a subclass called Car that overrides the drive() method to print "Repairing a car".
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics