Java Inheritance - Employee Class Hierarchy
Java Inheritance: Exercise-10 with Solution
Write a Java program that creates a class hierarchy for employees of a company. The base class should be Employee, with subclasses Manager, Developer, and Programmer. Each subclass should have properties such as name, address, salary, and job title. Implement methods for calculating bonuses, generating performance reports, and managing projects.
Sample Solution:
Java Code:
//Employee.java
class Employee {
private String name;
private String address;
private double salary;
private String jobTitle;
public Employee(String name, String address, double salary, String jobTitle) {
this.name = name;
this.address = address;
this.salary = salary;
this.jobTitle = jobTitle;
}
public String getName() {
return name;
}
public String getAddress() {
return address;
}
public double getSalary() {
return salary;
}
public String getJobTitle() {
return jobTitle;
}
public double calculateBonus() {
// Default implementation for bonus calculation
return 0.0;
}
public String generatePerformanceReport() {
// Default implementation for performance report
return "No performance report available.";
}
}
Explanation:
- Employee class: This class represents a generic employee with private instance variables 'name', 'address', 'salary', and 'jobTitle'. It also provides getter methods to access these private variables.
- getName(): Returns the employee's name.
- getAddress(): Returns the employee's address.
- getSalary(): Returns the employee's salary.
- getJobTitle(): Returns the employee's job title.
- calculateBonus(): This method is used to calculate the bonus for an employee. In the base class, it provides a default implementation that returns 0.0. Subclasses can override this method to provide custom bonus calculation logic.
- generatePerformanceReport(): This method generates a performance report for an employee. Similar to the bonus calculation, it provides a default implementation that returns "No performance report available." Subclasses can override this method to provide custom performance report generation logic.
This class is designed to be extended by subclasses like "Manager", "Developer", and "Programmer", which can provide their own implementations of bonus calculation and performance report generation as per their specific roles and responsibilities.
//Manager.java
class Manager extends Employee {
private int numberOfSubordinates;
public Manager(String name, String address, double salary, String jobTitle, int numberOfSubordinates) {
super(name, address, salary, jobTitle);
this.numberOfSubordinates = numberOfSubordinates;
}
public int getNumberOfSubordinates() {
return numberOfSubordinates;
}
@Override
public double calculateBonus() {
// Custom implementation for bonus calculation for managers
return getSalary() * 0.15;
}
@Override
public String generatePerformanceReport() {
// Custom implementation for performance report for managers
return "Performance report for Manager " + getName() + ": Excellent";
}
public void manageProject() {
// Custom method for managing projects
System.out.println("Manager " + getName() + " is managing a project.");
}
}
Explanation:
- extends Employee: This line indicates that the "Manager" class inherits from the "Employee" class. It means that a Manager is a specialized type of Employee and inherits all the attributes and methods of the Employee class.
- private int numberOfSubordinates: This instance variable represents the number of subordinates managed by the manager. It is specific to the "Manager" class and not present in the base "Employee" class.
- public Manager(String name, String address, double salary, String jobTitle, int numberOfSubordinates): This is the constructor for the "Manager" class. It takes parameters for 'name', 'address', 'salary', 'jobTitle', and numberOfSubordinates, which are used to initialize the attributes inherited from the "Employee" class as well as the numberOfSubordinates specific to managers. The super(...) keyword is used to call the constructor of the superclass (Employee) to initialize its attributes.
- public int getNumberOfSubordinates(): This method allows you to retrieve the number of subordinates managed by the manager.
- @Override public double calculateBonus(): This method is marked with the @Override annotation, indicating that it is an overridden method from the superclass (Employee). The "calculateBonus()" method provides a custom implementation for bonus calculation for managers. In this case, it calculates the bonus as 15% of the manager's salary.
- @Override public String generatePerformanceReport(): Similar to the "calculateBonus()" method, this method is also marked as an override and provides a custom implementation for generating a performance report for managers. It returns a specific performance report message for managers, including the manager's name and an "Excellent" rating.
- public void manageProject(): This is a custom method specific to the "Manager" class. It simulates the action of a manager managing a project by printing a message to the console.
//Developer.java
class Developer extends Employee {
private String programmingLanguage;
public Developer(String name, String address, double salary, String jobTitle, String programmingLanguage) {
super(name, address, salary, jobTitle);
this.programmingLanguage = programmingLanguage;
}
public String getProgrammingLanguage() {
return programmingLanguage;
}
@Override
public double calculateBonus() {
// Custom implementation for bonus calculation for developers
return getSalary() * 0.10;
}
@Override
public String generatePerformanceReport() {
// Custom implementation for performance report for developers
return "Performance report for Developer " + getName() + ": Good";
}
public void writeCode() {
// Custom method for writing code
System.out.println("Developer " + getName() + " is writing code in " + programmingLanguage);
}
}
Explanation:
- extends Employee: Similar to the "Manager" class, this line indicates that the Developer class inherits from the "Employee" class. It means that a 'Developer' is a specialized type of 'Employee' and inherits all the attributes and methods of the Employee class.
- private String programmingLanguage: This instance variable represents the programming language that the developer specializes in. It is specific to the "Developer" class and not present in the base "Employee" class.
- public Developer(String name, String address, double salary, String jobTitle, String programmingLanguage): This is the constructor for the "Developer" class. It takes parameters for 'name', 'address', 'salary', 'jobTitle', and programmingLanguage, which are used to initialize the attributes inherited from the "Employee" class as well as the programmingLanguage specific to developers. The super(...) keyword is used to call the constructor of the superclass (Employee) to initialize its attributes.
- public String getProgrammingLanguage(): This method allows you to retrieve the programming language specialization of the developer.
- @Override public double calculateBonus(): This method is marked with the @Override annotation, indicating that it is an overridden method from the superclass (Employee). The "calculateBonus()" method provides a custom implementation for bonus calculation for developers. In this case, it calculates the bonus as 10% of the developer's salary.
- @Override public String generatePerformanceReport(): Similar to the "calculateBonus()" method, this method is also marked as an override and provides a custom implementation for generating a performance report for developers. It returns a specific performance report message for developers, including the developer's name and a "Good" rating.
- public void writeCode(): This is a custom method specific to the "Developer" class. It simulates the action of a developer writing code in their specialized programming language by printing a message to the console.
//Programmer.java
class Programmer extends Developer {
public Programmer(String name, String address, double salary, String programmingLanguage) {
super(name, address, salary, "Programmer", programmingLanguage);
}
@Override
public double calculateBonus() {
// Custom implementation for bonus calculation for programmers
return getSalary() * 0.12;
}
@Override
public String generatePerformanceReport() {
// Custom implementation for performance report for programmers
return "Performance report for Programmer " + getName() + ": Excellent";
}
public void debugCode() {
// Custom method for debugging code
System.out.println("Programmer " + getName() + " is debugging code in " + getProgrammingLanguage());
}
}
Explanation:
- extends Developer: This line indicates that the "Programmer" class inherits from the "Developer" class. It means that a 'Programmer' is a specialized type of 'Developer' and inherits all the attributes and methods of the Developer class.
- public Programmer(String name, String address, double salary, String programmingLanguage): This is the constructor for the "Programmer" class. It takes parameters for 'name', 'address', 'salary', and 'programmingLanguage'. It passes these parameters to the constructor of the superclass (Developer) using the super(...) keyword to initialize the attributes inherited from the "Developer" class. The 'jobTitle' parameter is set to "Programmer" to indicate the specific job title for programmers.
- @Override public double calculateBonus(): This method is marked with the @Override annotation, indicating that it is an overridden method from the superclass (Developer). The "calculateBonus()" method provides a custom implementation for bonus calculation for programmers. In this case, it calculates the bonus as 12% of the programmer's salary.
- @Override public String generatePerformanceReport(): Similar to the "calculateBonus()" method, this method is also marked as an override and provides a custom implementation for generating a performance report for programmers. It returns a specific performance report message for programmers, including the programmer's name and an "Excellent" rating.
- public void debugCode(): This is a custom method specific to the "Programmer" class. It simulates the action of a programmer debugging code in their specialized programming language by printing a message to the console.
//Main.java
public class Main {
public static void main(String[] args) {
Manager manager = new Manager("Avril Aroldo", "1 ABC St", 80000.0, "Manager", 5);
Developer developer = new Developer("Iver Dipali", "2 PQR St", 72000.0, "Developer", "Java");
Programmer programmer = new Programmer("Yaron Gabriel", "3 ABC St", 76000.0, "Python");
System.out.println("Manager's Bonus: $" + manager.calculateBonus());
System.out.println("Developer's Bonus: $" + developer.calculateBonus());
System.out.println("Programmer's Bonus: $" + programmer.calculateBonus());
System.out.println(manager.generatePerformanceReport());
System.out.println(developer.generatePerformanceReport());
System.out.println(programmer.generatePerformanceReport());
manager.manageProject();
developer.writeCode();
programmer.debugCode();
}
}
Explanation:
- Creating Employee Objects:
- Three employee objects are created: 'manager', 'developer', and 'programmer', each with their specific attributes such as name, address, salary, and job title.
- manager is an instance of the "Manager" class.
- developer is an instance of the "Developer" class.
- programmer is an instance of the "Programmer" class.
- Calculating Bonuses:
- The program calls the "calculateBonus()" method for each employee type (manager, developer, and programmer) to calculate their respective bonuses.
- The bonuses are displayed on the console.
- Generating Performance Reports:
- The program calls the "generatePerformanceReport()" method for each employee type (manager, developer, and programmer) to generate performance reports.
- The performance reports are displayed on the console.
- Specific Actions:
- For each employee type, specific actions are performed:
- manager uses the "manageProject()" method to simulate managing a project.
- developer uses the "writeCode()" method to simulate writing code in a specific programming language.
- programmer uses the "debugCode()" method to simulate debugging code in a specific programming language.
- These actions are displayed on the console.
Sample Output:
Manager's Bonus: $12000.0 Developer's Bonus: $7200.0 Programmer's Bonus: $9120.0 Performance report for Manager Avril Aroldo: Excellent Performance report for Developer Iver Dipali: Good Performance report for Programmer Yaron Gabriel: Excellent Manager Avril Aroldo is managing a project. Developer Iver Dipali is writing code in Java Programmer Yaron Gabriel is debugging code in Python
Flowchart of Employee class:
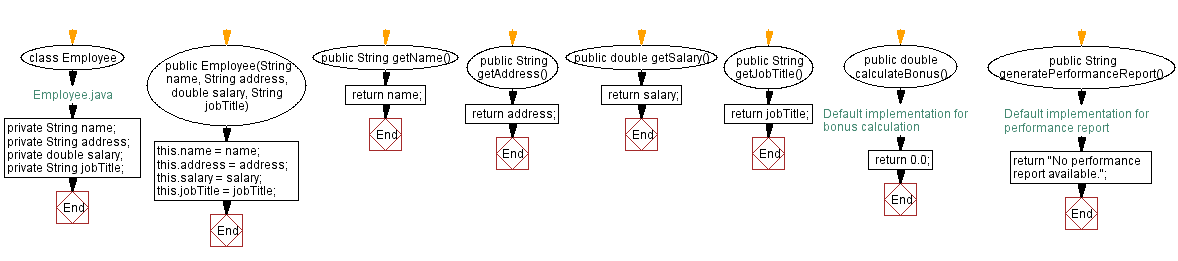
Flowchart of Manager class:
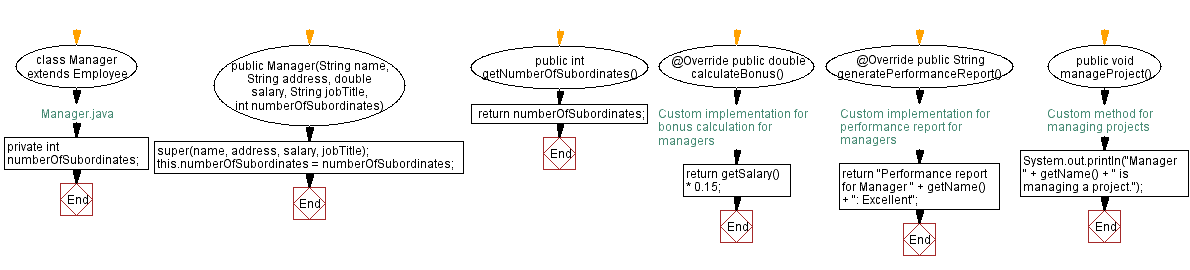
Flowchart of Developer class:
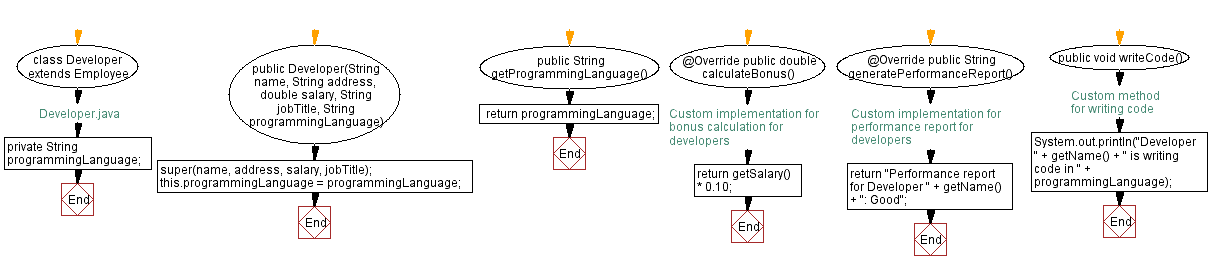
Flowchart of Programmer class:
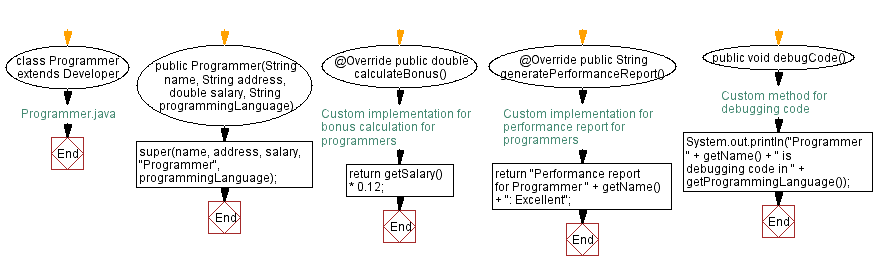
Flowchart of Main class:
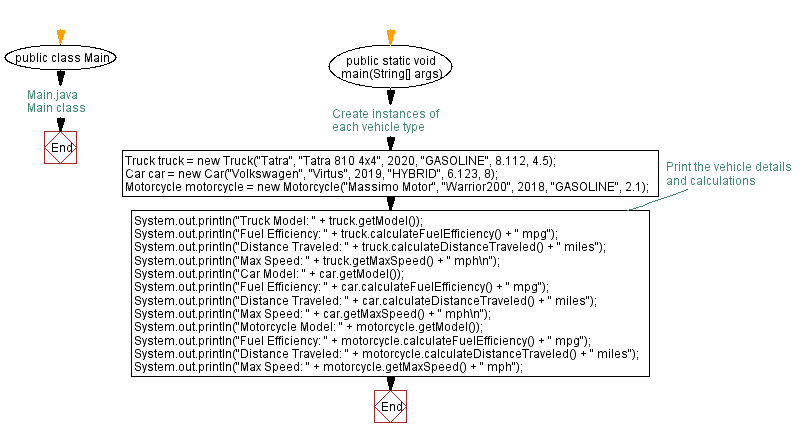
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Create a class called Shape with methods called getPerimeter() and getArea().
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics