Java Program: Drawable interface & shape implementations
Java Interface: Exercise-6 with Solution
Write a Java program to create an interface Drawable with a method draw() that takes no arguments and returns void. Create three classes Circle, Rectangle, and Triangle that implement the Drawable interface and override the draw() method to draw their respective shapes.
Sample Solution:
Java Code:
// Drawable.java
// Declare the Drawable interface
interface Drawable {
// Declare the abstract method "draw" that classes implementing this interface must provide
void draw();
}
// Circle.java
// Import necessary libraries for graphics and user interface
import java.awt.*;
import javax.swing.*;
// Declare the Circle class, which implements the Drawable interface
class Circle implements Drawable {
// Implement the "draw" method required by the Drawable interface
public void draw() {
// Create a new JFrame for displaying the circle
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setVisible(true);
// Create a JPanel for custom drawing
JPanel panel = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Set the drawing color to red and fill an oval
g.setColor(Color.RED);
g.fillOval(100, 100, 200, 200);
}
};
// Add the panel to the frame
frame.add(panel);
}
}
// Rectangle.java
// Import necessary libraries for graphics and user interface
import java.awt.*;
import javax.swing.*;
// Declare the Rectangle class, which implements the Drawable interface
class Rectangle implements Drawable {
// Implement the "draw" method required by the Drawable interface
public void draw() {
// Create a new JFrame for displaying the rectangle
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setVisible(true);
// Create a JPanel for custom drawing
JPanel panel = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Set the drawing color to blue and fill a rectangle
g.setColor(Color.BLUE);
g.fillRect(100, 100, 200, 200);
}
};
// Add the panel to the frame
frame.add(panel);
}
}
// Triangle.java
// Import necessary libraries for graphics and user interface
import java.awt.*;
import javax.swing.*;
// Declare the Triangle class, which implements the Drawable interface
class Triangle implements Drawable {
// Implement the "draw" method required by the Drawable interface
public void draw() {
// Create a new JFrame for displaying the triangle
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setVisible(true);
// Create a JPanel for custom drawing
JPanel panel = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Set the drawing color to green and fill a polygon
g.setColor(Color.GREEN);
int[] xPoints = {
200,
100,
300
};
int[] yPoints = {
100,
300,
300
};
g.fillPolygon(xPoints, yPoints, 3);
}
};
// Add the panel to the frame
frame.add(panel);
}
}
// Main.java
// Import necessary libraries for graphics and user interface
import java.awt.*;
import javax.swing.*;
// Declare the Main class
public class Main {
public static void main(String[] args) {
// Create instances of Drawable objects, which are Circle, Rectangle, and Triangle
Drawable circle = new Circle();
Drawable rectangle = new Rectangle();
Drawable triangle = new Triangle();
// Call the "draw" method to display each shape
circle.draw();
rectangle.draw();
triangle.draw();
}
}
Sample Output:
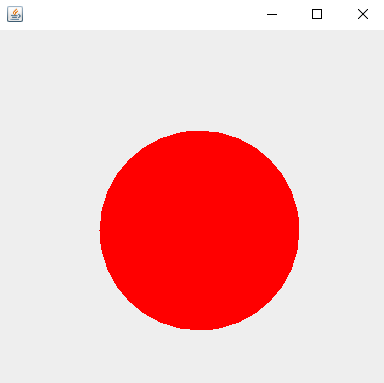
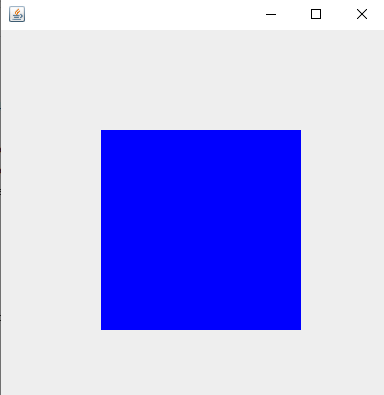
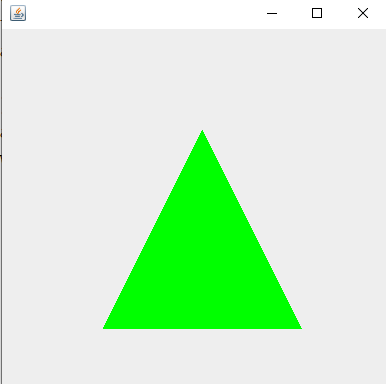
Explanation:
In the above exercise –
- The "Circle", "Rectangle", and "Triangle" classes implement the Drawable interface and provide their own implementations of the draw() method. Each class overrides the draw() method to print a message indicating the shape being drawn. It may also include code to draw the shape.
- In the main() method, we create instances of the Circle, Rectangle, and Triangle classes and assign them to variables of type Drawable. We then call the draw() method on each variable, which invokes the overridden implementation of the respective shape class.
Flowchart of Drawable Java:
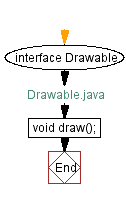
Flowchart of Circle Java:
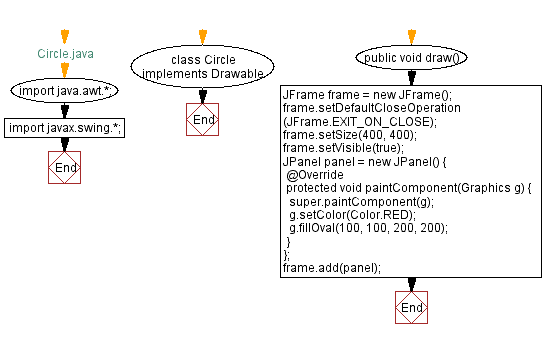
Flowchart of Rectangle Java:
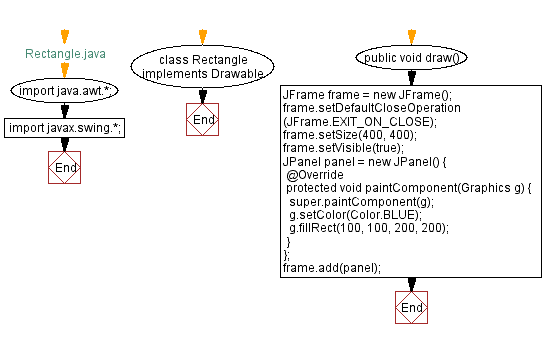
Flowchart of Triangle:
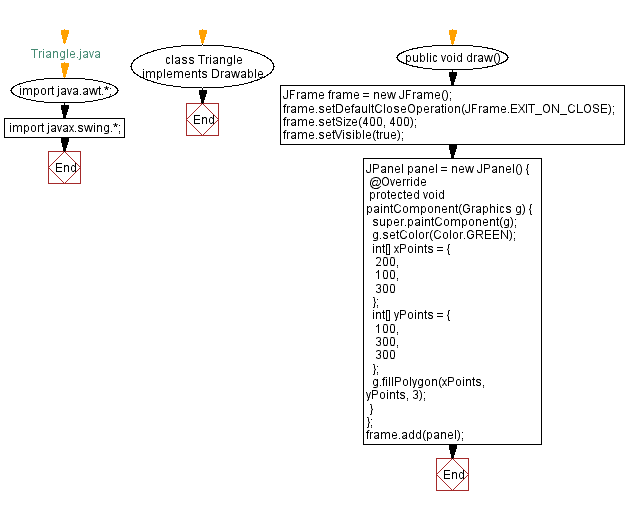
Flowchart of Main Java:
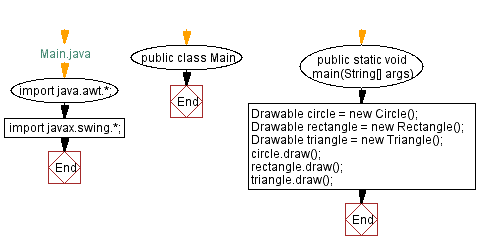
Java Code Editor:
Previous: Resizable interface for object resizing with rectangle class implementation.
Next: Sortable interface with bubbleSort and selectionSort implementations.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics