JavaFX Login form application
8. Login Form with Action Listener
Write a JavaFX login form. Implement an action listener on the "Login" button to validate user credentials.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Login Form");
GridPane grid = new GridPane();
grid.setHgap(10);
grid.setVgap(10);
grid.setPadding(new Insets(20, 20, 20, 20));
Label usernameLabel = new Label("Username:");
TextField usernameField = new TextField();
Label passwordLabel = new Label("Password:");
PasswordField passwordField = new PasswordField();
Button loginButton = new Button("Login");
loginButton.setOnAction(event -> {
String username = usernameField.getText();
String password = passwordField.getText();
// Replace with your authentication logic here
if (authenticate(username, password)) {
showAlert("Login Successful", "Welcome, " + username + "!");
} else {
showAlert("Login Failed", "Invalid username or password.");
}
});
grid.add(usernameLabel, 0, 0);
grid.add(usernameField, 1, 0);
grid.add(passwordLabel, 0, 1);
grid.add(passwordField, 1, 1);
grid.add(loginButton, 1, 2);
Scene scene = new Scene(grid, 300, 150);
primaryStage.setScene(scene);
primaryStage.show();
}
private boolean authenticate(String username, String password) {
// Replace with your authentication logic (e.g., database check)
return username.equals("admin") && password.equals("pass123$");
}
private void showAlert(String title, String message) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setContentText(message);
alert.showAndWait();
}
}
Sample Output:
Flowchart:
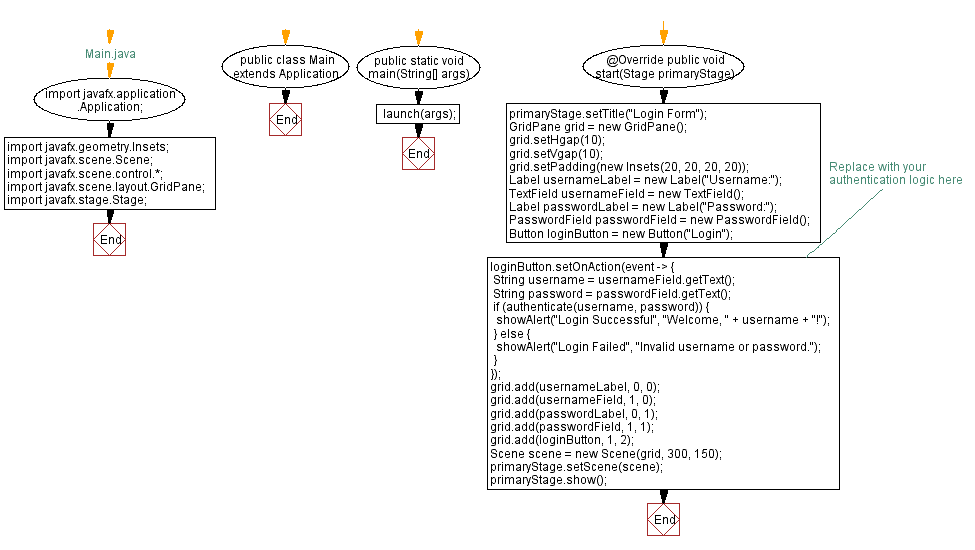
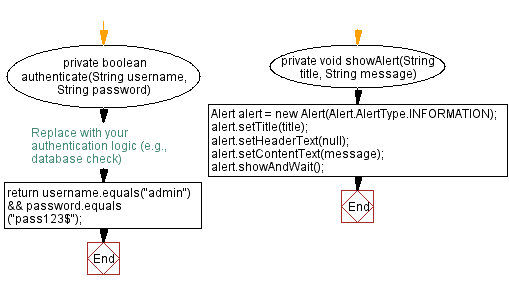
Go to:
PREV : Shopping Cart with Item Buttons.
NEXT : Nested Panes Event Filtering.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.