Java Program: Lambda expression to convert integer to binary
Java Lambda Program: Exercise-25 with Solution
Write a Java program to implement a lambda expression to convert an integer to their corresponding binary representation.
Sample Solution:
Java Code:
import java.util.function.Function;
public class Main {
public static void main(String[] args) {
int n = 33;
System.out.println("Number: " + n);
Function < Integer, String > convertToBinary = num -> Integer.toBinaryString(num);
String binaryRepresentation = convertToBinary.apply(n);
System.out.println("Binary representation: " + binaryRepresentation);
n = 747;
System.out.println("\nNumber: " + n);
convertToBinary = num -> Integer.toBinaryString(num);
binaryRepresentation = convertToBinary.apply(n);
System.out.println("Binary representation: " + binaryRepresentation);
}
}
Sample Output:
Number: 33 Binary representation: 100001 Number: 747 Binary representation: 1011101011
Explanation:
In the above exercise the program uses the Integer.toBinaryString() method to convert an integer to its binary representation. The lambda expression allows you to encapsulate this conversion logic and use it as a function.
The lambda expression:
- It takes an integer as input (num).
- It uses the Integer.toBinaryString() method to convert the integer to its binary representation.
- It returns the binary representation as a string.
Flowchart:
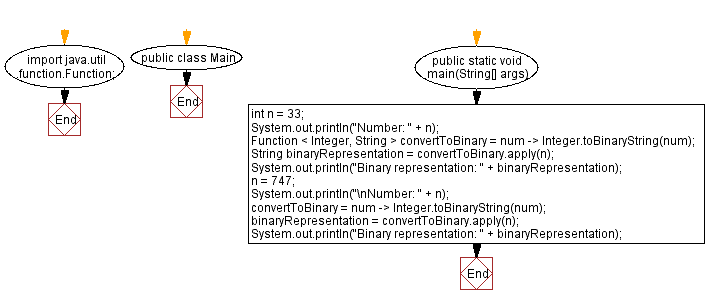
Live Demo:
Java Code Editor:
Improve this sample solution and post your code through Disqus
Java Lambda Exercises Previous: Lambda expression to find largest prime factor.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/lambda/java-lambda-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics