Java: Calculate power of a number without using multiplication and division operators
Java Math Exercises: Exercise-16 with Solution
Power Without Multiplication or Division
Write a Java program to calculate power of a number without using multiplication(*) and division(/) operators.
Sample Solution:
Java Code:
import java.util.*;
public class solution {
public static int power(int b, int e)
{
if (e == 0)
return 1;
int result = b;
int temp = b;
int i, j;
for (i = 1; i < e; i++) {
for (j = 1; j < b; j++) {
result += temp;
}
temp = result;
}
return result;
}
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.print("Input the base: ");
int b = scan.nextInt();
System.out.print("Input the exponent: ");
int e = scan.nextInt();
scan.close();
if (b>0 && e>0)
System.out.println("Power of the number: "+power(b, e));
}
}
Sample Output:
Input the base: 2 Input the exponent: 5 Power of the number: 32
Flowchart:
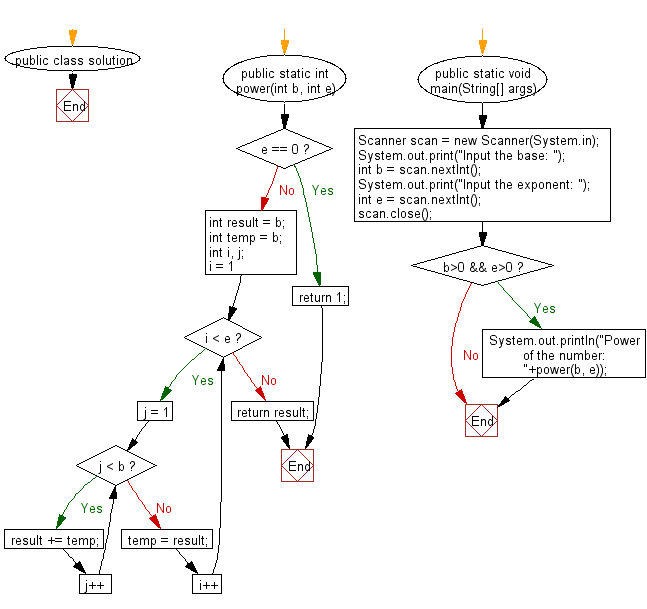
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Next: Write a Java program to calculate and print average (or mean) of the stream of given numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/math/java-math-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics