Java: Calculate the area of a triangle
Calculate Triangle Area
Write Java methods to calculate triangle area.
Pictorial Presentation:
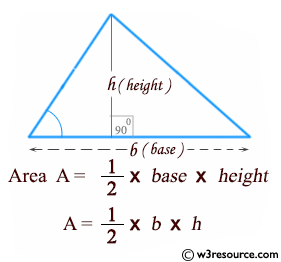
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise13 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input Side-1: ");
double side1 = in.nextDouble();
System.out.print("Input Side-2: ");
double side2 = in.nextDouble();
System.out.print("Input Side-3: ");
double side3 = in.nextDouble();
System.out.println( is_Valid(side1, side2,side3) ?
"The area of the triangle is " + area_triangle(side1, side2, side3) : "Invalid triangle" );
}
public static boolean is_Valid(double side1, double side2, double side3) {
if( side1 + side2 > side3 &&
side2 + side3 > side1 &&
side1 + side3 > side2) return true;
else return false;
}
public static double area_triangle(double side1, double side2, double side3) {
double area = 0;
double s = (side1 + side2 + side3)/2;
area = Math.sqrt(s*(s - side1)*(s - side2)*(s - side3));
return area;
}
}
Sample Output:
Input Side-1: 10 Input Side-2: 15 Input Side-3: 20 The area of the triangle is 72.61843774138907
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Java program to calculate the area of a triangle using Heron’s formula given its three side lengths.
- Write a Java program to compute the area of a right triangle using base and height inputs.
- Write a Java program to calculate the area of a triangle using the sine of an angle given two sides and the included angle.
- Write a Java program to compute the area of an equilateral triangle given its side length.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java method (takes a number n as input) to displays an n-by-n matrix.
Next: Write a Java method to create the area of a pentagon.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.