Java: Extract the first digit from an integer
Extract First Digit of Integer
Write a Java method for extracting the first digit from a positive or negative integer.
Pictorial Presentation:
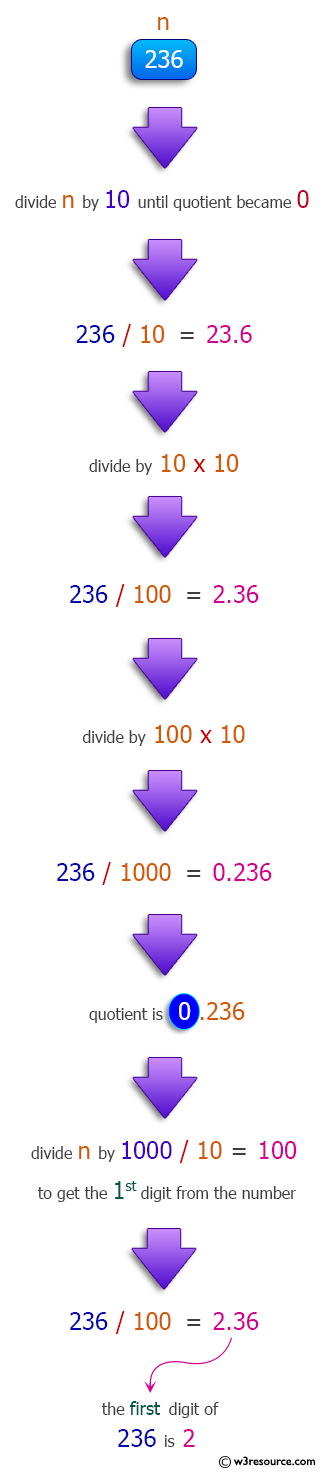
Sample data:
(1234) ->1
(-2345) ->2
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input an integer(positive/negative):");
int n = in.nextInt();
System.out.print("Extract the first digit from the said integer:\n");
System.out.print(test(n));
}
public static int test(int n){
int fact_num = 10;
while(n / fact_num != 0){
fact_num *= 10;
}
return Math.abs(n / (fact_num/10));
}
}
Sample Output:
Input an integer(positive/negative): 1234 Extract the first digit from the said integer: 1
Flowchart :
For more Practice: Solve these Related Problems:
- Write a Java program to extract the first digit of an integer using logarithmic operations.
- Write a Java program to extract the first digit of an integer without converting it to a string.
- Write a Java program to extract the first digit and then remove it from the given integer.
- Write a Java program to extract the first non-zero digit of an integer that may have leading zeros.
Go to:
PREV : Check If One Integer is Midpoint of Others.
NEXT : Display Factors of 3 in Integer
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.