Java: Count all words in a string
Count Words in String
Write a Java method to count all the words in a string.
Test Data:
Input the string: The quick brown fox jumps over the lazy
dog.
Pictorial Presentation:
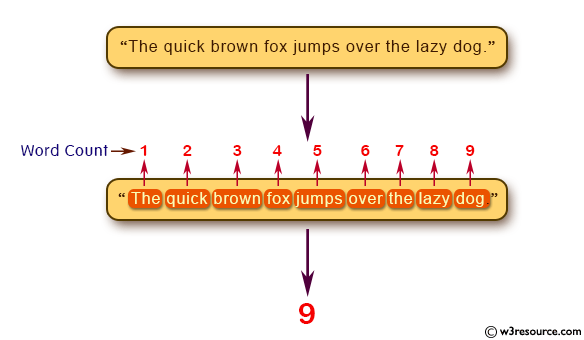
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise5 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input the string: ");
String str = in.nextLine();
System.out.print("Number of words in the string: " + count_Words(str)+"\n");
}
public static int count_Words(String str)
{
int count = 0;
if (!(" ".equals(str.substring(0, 1))) || !(" ".equals(str.substring(str.length() - 1))))
{
for (int i = 0; i < str.length(); i++)
{
if (str.charAt(i) == ' ')
{
count++;
}
}
count = count + 1;
}
return count; // returns 0 if string starts or ends with space " ".
}
}
Sample Output:
Input the string: The quick brown fox jumps over the lazy dog Number of words in the string: 9
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Java program to count the number of words in a string without using the split() method.
- Write a Java program to count words in a string by treating punctuation marks as additional delimiters.
- Write a Java program to trim extra spaces from a string and then count the number of words.
- Write a Java program to count words in a string and then output the longest word found.
Go to:
PREV : Count Vowels in String.
NEXT : Sum of Digits in Integer.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.