Java: Create and Modify Dog Objects
Java OOP: Exercise-2 with Solution
Write a Java program to create a class called "Dog" with a name and breed attribute. Create two instances of the "Dog" class, set their attributes using the constructor and modify the attributes using the setter methods and print the updated values.
Sample Solution:
Java Code:
// Define the Dog class
public class Dog {
// Declare a private variable to store the name of the dog
private String name;
// Declare a private variable to store the breed of the dog
private String breed;
// Constructor for the Dog class that initializes the name and breed variables
public Dog(String name, String breed) {
// Set the name variable to the provided name parameter
this.name = name;
// Set the breed variable to the provided breed parameter
this.breed = breed;
}
// Method to retrieve the name of the dog
public String getName() {
// Return the value of the name variable
return name;
}
// Method to set the name of the dog
public void setName(String name) {
// Set the name variable to the provided name parameter
this.name = name;
}
// Method to retrieve the breed of the dog
public String getBreed() {
// Return the value of the breed variable
return breed;
}
// Method to set the breed of the dog
public void setBreed(String breed) {
// Set the breed variable to the provided breed parameter
this.breed = breed;
}
}
The above class has two private attributes: ‘name’ and ‘breed’, and a constructor that initializes these attributes with the values passed as arguments. It also has getter and setter methods to access and modify these attributes.
// Define the Main class
public class Main {
// Define the main method which is the entry point of the program
public static void main(String[] args) {
// Create an instance of the Dog class with the name "Buddy" and breed "Golden Retriever"
Dog dog1 = new Dog("Buddy", "Golden Retriever");
// Create another instance of the Dog class with the name "Charlie" and breed "Bulldog"
Dog dog2 = new Dog("Charlie", "Bulldog");
// Print the name and breed of dog1 to the console
System.out.println(dog1.getName() + " is a " + dog1.getBreed() + ".");
// Print the name and breed of dog2 to the console
System.out.println(dog2.getName() + " is a " + dog2.getBreed() + ".");
// Print a message indicating that the breed of dog1 and the name of dog2 will be changed
System.out.println("\nSet the new Breed of dog1 and new name of dog2:");
// Change the breed of dog1 to "Labrador Retriever"
dog1.setBreed("Labrador Retriever");
// Change the name of dog2 to "Daisy"
dog2.setName("Daisy");
// Print the updated name and breed of dog1 to the console
System.out.println(dog1.getName() + " is now a " + dog1.getBreed() + ".");
// Print the updated name and breed of dog2 to the console
System.out.println(dog2.getName() + " is now a " + dog2.getBreed() + ".");
}
}
In the above example code, we create two instances of the "Dog" class, set their attributes through the constructor, and print their name and breed using the getter methods. We also modify the attributes using the setter methods and print the updated values.
Sample Output:
Buddy is a Golden Retriever. Charlie is a Bulldog. Set the new Breed of dog1 and new name of dog2: Buddy is now a Labrador Retriever. Daisy is now a Bulldog.
Flowchart:
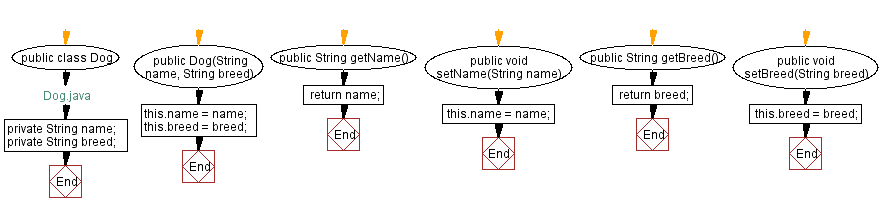
Java Code Editor:
Improve this sample solution and post your code through Disqus.
Java OOP Previous: Create and print Person objects.
Java OOP Next: Calculate area and perimeter of a rectangle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/oop/java-oop-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics