Java: Traffic Light Simulation
Java OOP: Exercise-8 with Solution
Write a Java program to create class called "TrafficLight" with attributes for color and duration, and methods to change the color and check for red or green.
Sample Solution:
Java Code:
//TrafficLight.java
// Define the TrafficLight class
public class TrafficLight {
// Declare a private variable to store the color of the traffic light
private String color;
// Declare a private variable to store the duration of the traffic light in seconds
private int duration;
// Constructor for the TrafficLight class
public TrafficLight(String color, int duration) {
// Initialize the color of the traffic light
this.color = color;
// Initialize the duration of the traffic light
this.duration = duration;
}
// Method to change the color of the traffic light
public void changeColor(String newColor) {
// Update the color variable to the new color
color = newColor;
}
// Method to check if the traffic light is red
public boolean isRed() {
// Return true if the color is red, otherwise return false
return color.equals("red");
}
// Method to check if the traffic light is green
public boolean isGreen() {
// Return true if the color is green, otherwise return false
return color.equals("green");
}
// Method to get the duration of the traffic light
public int getDuration() {
// Return the duration value
return duration;
}
// Method to set the duration of the traffic light
public void setDuration(int duration) {
// Update the duration variable to the new value
this.duration = duration;
}
}
The above class has two private attributes: ‘color’ and ‘duration’. A constructor initializes these attributes with the values passed as arguments, and getter and setter methods to access and modify these attributes. It also has methods to change the ‘color’, and to check if the light is red or green.
//Main.java
// Define the Main class
public class Main {
// Main method, the entry point of the Java application
public static void main(String[] args) {
// Create a new TrafficLight object with initial color "red" and duration 30 seconds
TrafficLight light = new TrafficLight("red", 30);
// Print whether the traffic light is red
System.out.println("The light is red: " + light.isRed());
// Print whether the traffic light is green
System.out.println("The light is green: " + light.isGreen());
// Change the color of the traffic light to "green"
light.changeColor("green");
// Print whether the traffic light is now green
System.out.println("The light is now green: " + light.isGreen());
// Print the duration of the traffic light
System.out.println("The light duration is: " + light.getDuration());
// Set a new duration for the traffic light to 20 seconds
light.setDuration(20);
// Print the updated duration of the traffic light
System.out.println("The light duration is now: " + light.getDuration());
}
}
In the above example code, we create an instance of the "TrafficLight" class with the color "red" and a duration of 30 seconds. We then print whether the light is red or green using the “isRed” and “isGreen” methods. We change the light color to "green" through the “changeColor” method, and display whether the light is now green. We also print the light duration using the “getDuration” method, and change the duration to 60 seconds with the “setDuration” method.
Sample Output:
The light is red: true The light is green: false The light is now green: true The light duration is: 30 The light duration is now: 20
Flowchart:
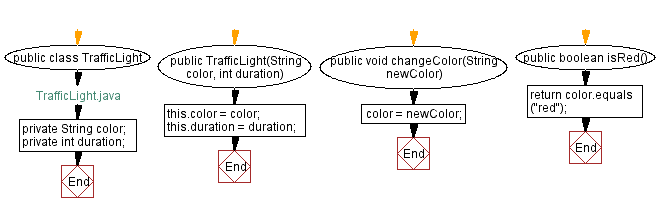
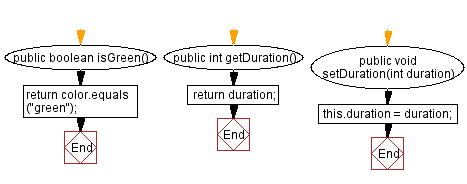
Java Code Editor:
Improve this sample solution and post your code through Disqus.
Java OOP Previous: Bank Account Management.
Java OOP Next: Employee class with years of service calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/oop/java-oop-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics