Java Recursive Method: Calculate the product of numbers in an array
Java Recursive: Exercise-13 with Solution
Recursive Product of Array Elements
Write a Java recursive method to calculate the product of all numbers in an array.
Sample Solution:
Java Code:
public class ArrayProductCalculator {
public static int calculateProduct(int[] arr) {
return calculateProduct(arr, 0, arr.length - 1);
}
private static int calculateProduct(int[] arr, int left, int right) {
// Base case: if the left and right indices are equal,
// return the single element as the product
if (left == right) {
return arr[left];
}
// Recursive case: divide the array into two halves, recursively
// calculate the product in each half,and return the product of
//the two calculated products
int mid = (left + right) / 2;
int productLeft = calculateProduct(arr, left, mid);
int productRight = calculateProduct(arr, mid + 1, right);
return productLeft * productRight;
}
public static void main(String[] args) {
int[] array = {
1,
3,
5,
7
};
int product = calculateProduct(array);
System.out.println("The product of all numbers in the array is: " + product);
}
}
Sample Output:
The product of all numbers in the array is: 105
Explanation:
In the above exercises -
We define a class "ArrayProductCalculator" that includes a recursive method calculateProduct() to calculate the product of all numbers in an array.
The calculateProduct() method has two cases:
- Base case: If the left and right indices are equal, we have a single element and return it as the product.
- Recursive case: For any array with more than one element, we divide the array into two halves by finding the middle index. We then recursively calculate the product in each half by calling the method with the appropriate indices. Finally, we return the product of the two calculated products obtained from the recursive calls.
In the main() method, we demonstrate the calculateProduct() method by calculating the product of all numbers in the array [1, 3, 5, 7] and printing the result.
Flowchart:
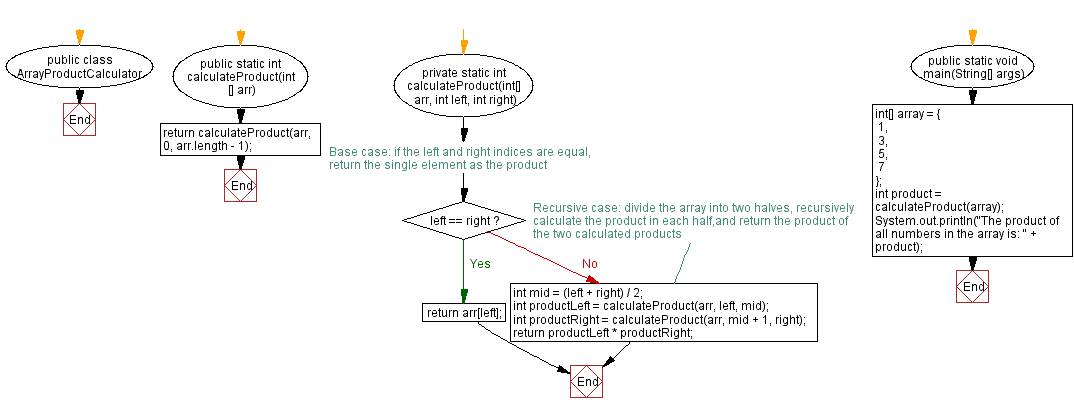
Java Code Editor:
Java Recursive Previous: Find the maximum element.
Java Recursive Next: Find the sum of digits in an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/recursive/java-recursive-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics