Java Recursive Method: String palindrome detection
Recursive String Palindrome Check
Write a Java recursive method to check if a given string is a palindrome.
Sample Solution:
Java Code:
public class PalindromeChecker {
public static boolean isPalindrome(String str) {
// Base case: an empty string or a string with one character is a palindrome
if (str.length() <= 1) {
return true;
}
// Recursive case: check if the first and last characters are equal,
// and recursively check if the substring between them is a palindrome
char firstChar = str.charAt(0);
char lastChar = str.charAt(str.length() - 1);
if (firstChar != lastChar) {
return false;
}
String remainingSubstring = str.substring(1, str.length() - 1);
return isPalindrome(remainingSubstring);
}
public static void main(String[] args) {
String palindrome1 = "madam";
boolean isPalindrome1 = isPalindrome(palindrome1);
System.out.println(palindrome1 + " is a palindrome: " + isPalindrome1);
String palindrome2 = "level";
boolean isPalindrome2 = isPalindrome(palindrome2);
System.out.println(palindrome2 + " is a palindrome: " + isPalindrome2);
String notPalindrome = "java";
boolean isPalindrome3 = isPalindrome(notPalindrome);
System.out.println(notPalindrome + " is a palindrome: " + isPalindrome3);
}
}
Sample Output:
madam is a palindrome: true level is a palindrome: true java is a palindrome: false
Explanation:
In the above exercises -
We define a class "PalindromeChecker" that includes a recursive method isPalindrome() to check if a given string str is a palindrome.
The isPalindrome() method has two cases:
- Base case: If the string length is 0 or 1, it returns true because an empty string or a string with one character is considered a palindrome.
- Recursive case: It compares the first and last characters of the string. If they are not equal, it returns false. Otherwise, it extracts the remaining substring between the first and last characters, and recursively checks if this substring is a palindrome. This process continues until the string is reduced to an empty string or one character.
In the main() method, we demonstrate the isPalindrome() method by checking if different strings are palindromes and printing the results.
Flowchart:
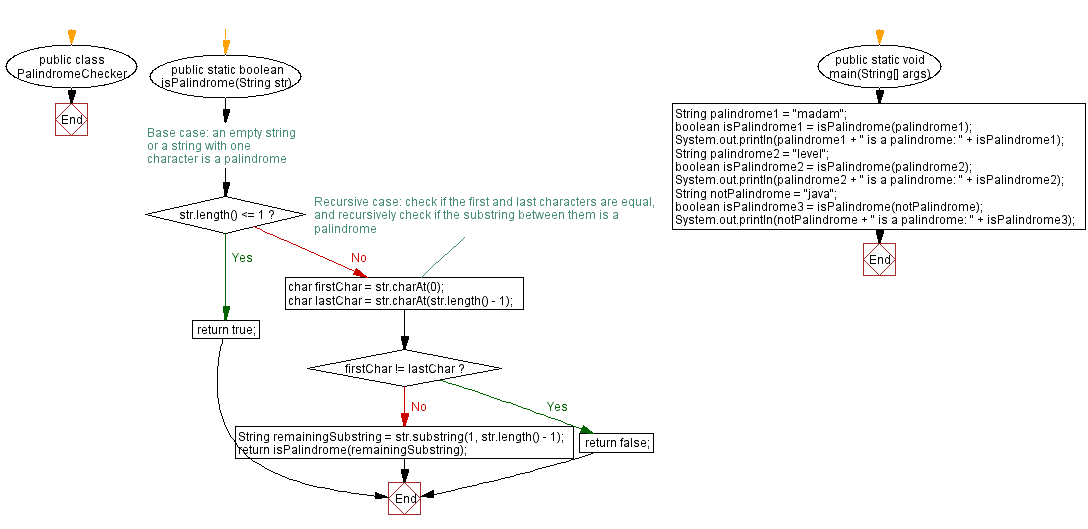
For more Practice: Solve these Related Problems:
- Write a Java program to recursively check if a string is a palindrome while ignoring case and non-alphanumeric characters.
- Write a Java program to implement a recursive palindrome check without using the substring method.
- Write a Java program to recursively compare characters from both ends of the string using a two-pointer technique.
- Write a Java program to recursively verify if a string is a mirrored palindrome based on a custom mapping of characters.
Java Code Editor:
Java Recursive Previous: Calculate the nth Fibonacci number.
Java Recursive Next: Calculate Base to Power.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.