Java: Pancake sort Algorithm
14. Pancake Sort Implementation
Write a Java program to sort an array of given integers using the Pancake sort algorithm.
Pancake sorting is the colloquial term for the mathematical problem of sorting a disordered stack of pancakes in order of size. This is when a spatula can be inserted at any point in the stack and used to flip all pancakes above it. Pancake numbers are the minimum number of flips required for a given number of pancakes. The problem was first discussed by American geometer Jacob E. Goodman. It is a variation of the sorting problem in which the only allowed operation is to reverse the elements of some prefix of the sequence.
Sample Solution:
Java Code:
import java.util.Arrays;
public class PancakeSort
{
int[] heap;
public String toString() {
String info = "";
for (int x: heap)
info += x + " ";
return info;
}
public void flip(int n) {
for (int i = 0; i < (n+1) / 2; ++i) {
int tmp = heap[i];
heap[i] = heap[n-i];
heap[n-i] = tmp;
}
// System.out.println("flip(0.." + n + "): " + toString());
}
public int[] minmax(int n) {
int xm, xM;
xm = xM = heap[0];
int posm = 0, posM = 0;
for (int i = 1; i < n; ++i) {
if (heap[i] < xm) {
xm = heap[i];
posm = i;
}
else if (heap[i] > xM) {
xM = heap[i];
posM = i;
}
}
return new int[] {posm, posM};
}
public void sort(int n, int dir) {
if (n == 0) return;
int[] mM = minmax(n);
int bestXPos = mM[dir];
int altXPos = mM[1-dir];
boolean flipped = false;
if (bestXPos == n-1) {
--n;
}
else if (bestXPos == 0) {
flip(n-1);
--n;
}
else if (altXPos == n-1) {
dir = 1-dir;
--n;
flipped = true;
}
else {
flip(bestXPos); }
sort(n, dir);
if (flipped) {
flip(n);
}
}
PancakeSort(int[] numbers) {
heap = numbers;
sort(numbers.length, 1);
}
public static void main(String[] args) {
int numbers[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(numbers));
PancakeSort pancakes = new PancakeSort(numbers);
System.out.println("Sorted Array:");
System.out.println(Arrays.toString(numbers));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array: [-5, 0, 1, 2, 3, 7, 45]]
Flowchart:
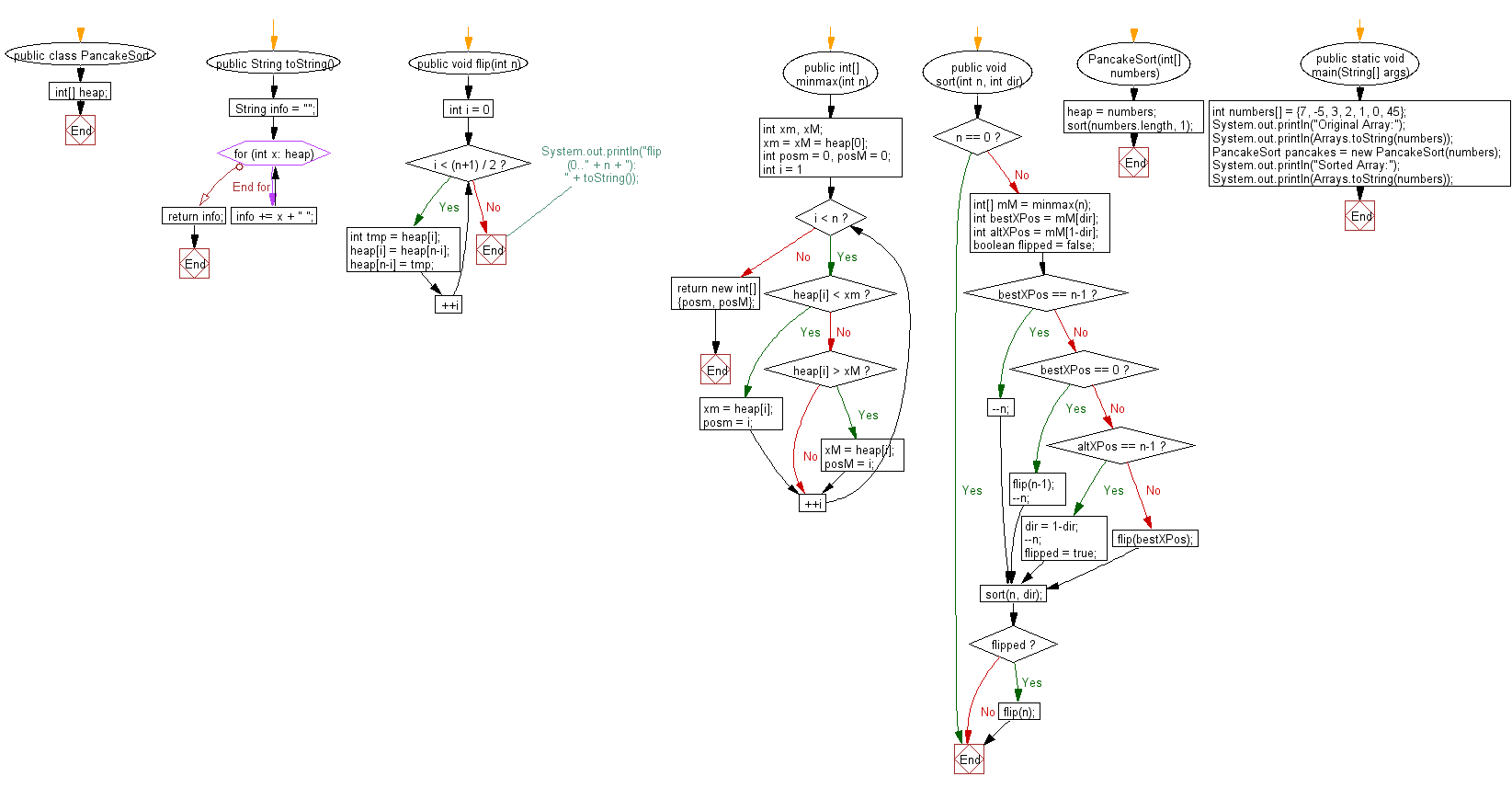
For more Practice: Solve these Related Problems:
- Write a Java program to implement pancake sort and count the number of flips required to sort the array.
- Write a Java program to modify pancake sort to sort an array of strings based on length.
- Write a Java program to implement pancake sort and optimize the flip process to reduce unnecessary reversals.
- Write a Java program to simulate pancake sort visually by printing the array state after each flip.
Go to:
PREV : Gnome Sort Implementation.
NEXT : Permutation Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.