Java: Top and bottom elements of a stack
Java Stack: Exercise-10 with Solution
Write a Java program to find the top and bottom elements of a given stack.
Sample Solution:
Java Code:
import java.util.Scanner;
import java.util.HashSet;
public class Stack {
private int[] arr;
private int top;
// Constructor to initialize the stack
public Stack(int size) {
arr = new int[size];
top = -1;
}
// Method to push an element onto the stack
public void push(int num) {
if (top == arr.length - 1) {
System.out.println("Stack is full");
} else {
top++;
arr[top] = num;
}
}
// Method to pop an element from the stack
public int pop() {
if (top == -1) {
System.out.println("Stack Underflow");
return -1;
} else {
int poppedElement = arr[top];
top--;
return poppedElement;
}
}
// Method to get the top element of the stack
public int peek() {
if (top == -1) {
System.out.println("Stack is empty");
return -1;
} else {
return arr[top];
}
}
// Method to check if the stack is empty
public boolean isEmpty() {
return top == -1;
}
// Method to remove duplicates
public void display() {
if (top == -1) {
System.out.println("Stack is empty");
} else {
System.out.print("Stack elements: ");
for (int i = top; i >= 0; i--) {
System.out.print(arr[i] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
System.out.println("Initialize a stack:");
Stack stack = new Stack(10);
System.out.println("\nInput some elements on the stack:");
stack.push(1);
stack.push(3);
stack.push(2);
stack.push(0);
stack.push(7);
stack.push(5);
stack.push(-1);
stack.push(3);
stack.push(-1);
stack.display();
System.out.println("\nGet the top element:");
int top_element = stack.peek();
System.out.println("Top element: " + top_element);
System.out.println("\nGet the bottom element:");
int bottom_element = -1;
while (!stack.isEmpty()) {
bottom_element = stack.pop();
}
System.out.println("Bottom element: " + bottom_element);
}
}
Sample Output:
Initialize a stack: Input some elements on the stack: Stack elements: -1 3 -1 5 7 0 2 3 1 Get the top element: Top element: -1 Get the bottom element: Bottom element: 1
Flowchart:
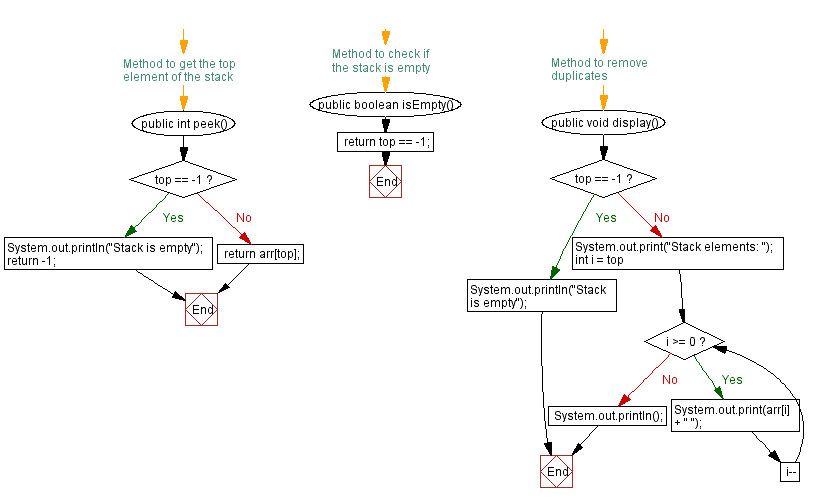
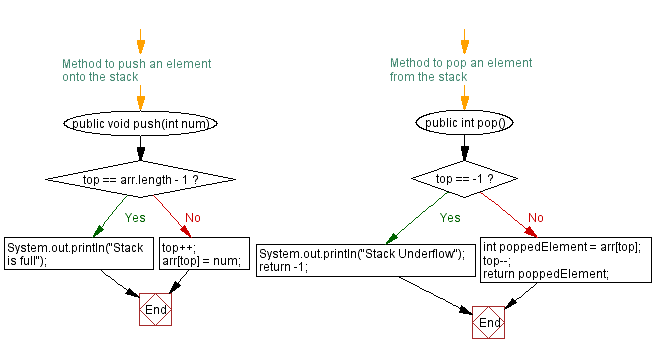
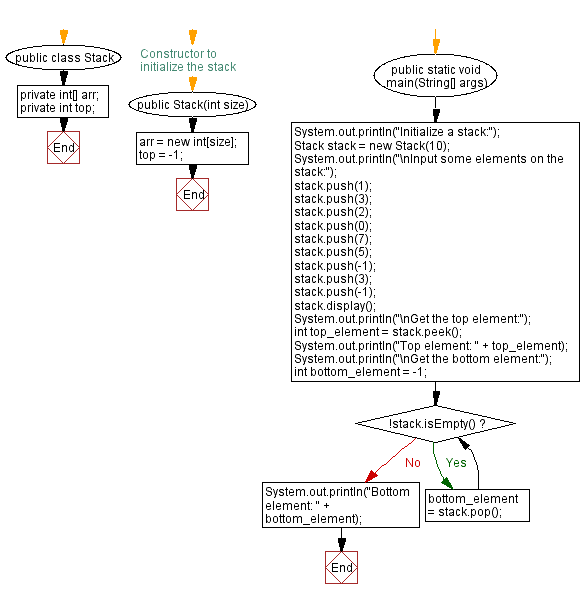
Live Demo:
Java Code Editor:
Improve this sample solution and post your code through Disqus
Java Stack Previous: Remove duplicates from a given stack.
Java Stack Exercises Next: Rotate the stack elements to the right.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics