Java Program: Sort List of Strings in Ascending and Descending Order using Streams
6. Sort strings A-Z and Z-A using streams
Write a Java program to sort a list of strings in alphabetical order, ascending and descending using streams.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List < String > colors = Arrays.asList("Red", "Green", "Blue", "Pink", "Brown");
System.out.println("Original List of strings(colors): " + colors);
// Sort strings in ascending order
List < String > ascendingOrder = colors.stream()
.sorted()
.collect(Collectors.toList());
// Sort strings in descending order
List < String > descendingOrder = colors.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
System.out.println("\nSorted in Ascending Order: " + ascendingOrder);
System.out.println("\nSorted in Descending Order: " + descendingOrder);
}
}
Sample Output:
Original List of strings(colors): [Red, Green, Blue, Pink, Brown] Sorted in Ascending Order: [Blue, Brown, Green, Pink, Red] Sorted in Descending Order: [Red, Pink, Green, Brown, Blue]
Explanation:
In the above exercise,
Using streams, we call the sorted() method without passing any comparator to sort the strings in ascending order based on their natural order (lexicographically). We collect the sorted strings into a new list using the toList() method.
To sort the strings in descending order, we pass Comparator.reverseOrder() as the comparator to the sorted() method. This reverses the natural order and sorts the strings in descending order.
Flowchart:
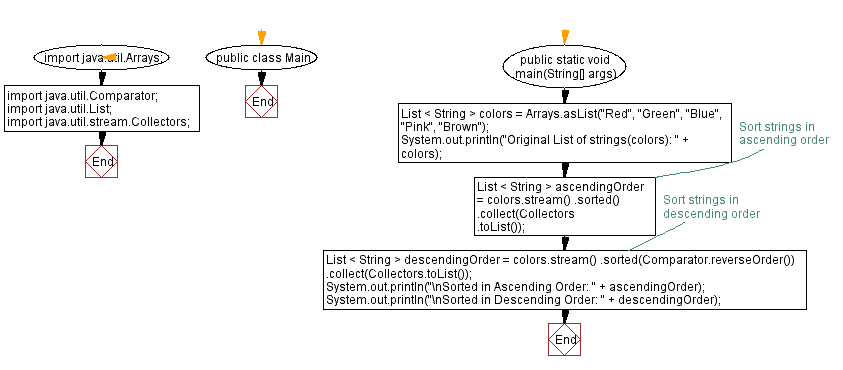
For more Practice: Solve these Related Problems:
- Write a Java program to sort a list of strings in ascending order using streams and then reverse the order to get descending order.
- Write a Java program to sort a list of strings by their last character in ascending order using streams.
- Write a Java program to sort a list of strings in descending order ignoring case using streams.
- Write a Java program to sort a list of strings first by their length and then alphabetically using streams.
Go to:
PREV : Count strings starting with letter using streams.
NEXT : Find max and min in list using streams.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.