Java Program: Find Second Smallest and Largest Elements in a List of Integers using Streams
8. Find 2nd smallest/largest using streams
Write a Java program to find the second smallest and largest elements in a list of integers using streams.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List < Integer > nums = Arrays.asList(1, 17, 54, 14, 14, 33, 45, -11);
System.out.println("List of numbers: " + nums);
// Find the second smallest element
Integer secondSmallest = nums.stream()
.distinct()
.sorted()
.skip(1)
.findFirst()
.orElse(null);
// Find the second largest element
Integer secondLargest = nums.stream()
.distinct()
.sorted((a, b) -> Integer.compare(b, a))
.skip(1)
.findFirst()
.orElse(null);
System.out.println("\nSecond smallest element: " + secondSmallest);
System.out.println("\nSecond largest element: " + secondLargest);
}
}
Sample Output:
List of numbers: [1, 17, 54, 14, 14, 33, 45, -11] Second smallest element: 1 Second largest element: 45
Explanation:
In the above exercise,
We create a list of integers (numbers) and use streams to find the second smallest element by applying distinct, sorting the elements in ascending order, skipping the first element, and locating the first element. Similarly, we find the second largest element by sorting the elements in descending order. The orElse method handles the case when the list is empty or there are no second smallest/largest elements.
Flowchart:
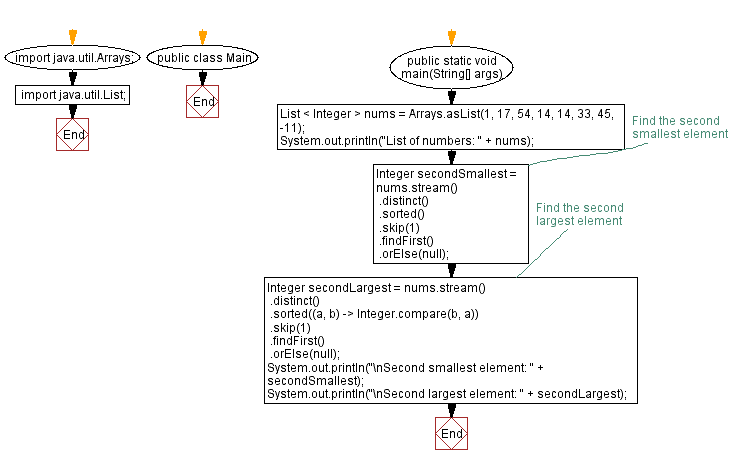
For more Practice: Solve these Related Problems:
- Write a Java program to find the second smallest and second largest values in a list of integers using streams after sorting.
- Write a Java program to extract the second smallest and second largest numbers from a list by first removing duplicates using streams.
- Write a Java program to compute the second smallest and second largest numbers by mapping a list to a sorted stream and then using skip().
- Write a Java program to find the second smallest and second largest elements in a list using streams and then calculate their sum.
Go to:
PREV : Find max and min in list using streams.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.