Java: Compare a given string to the specified string buffer
Java String: Exercise-10 with Solution
Write a Java program to compare a given string to a specified string buffer.
Sample Solution:
Java Code:
// Define a public class named Exercise10.
public class Exercise10 {
// Define the main method.
public static void main(String[] args) {
// Declare and initialize two string variables, str1 and str2.
String str1 = "example.com", str2 = "Example.com";
// Create a StringBuffer object strbuf initialized with the value of str1.
StringBuffer strbuf = new StringBuffer(str1);
// Compare str1 and strbuf for content equality and print the result.
System.out.println("Comparing " + str1 + " and " + strbuf + ": " + str1.contentEquals(strbuf));
// Compare str2 and strbuf for content equality and print the result.
System.out.println("Comparing " + str2 + " and " + strbuf + ": " + str2.contentEquals(strbuf));
}
}
Sample Output:
Comparing example.com and example.com: true Comparing Example.com and example.com: false
Flowchart:
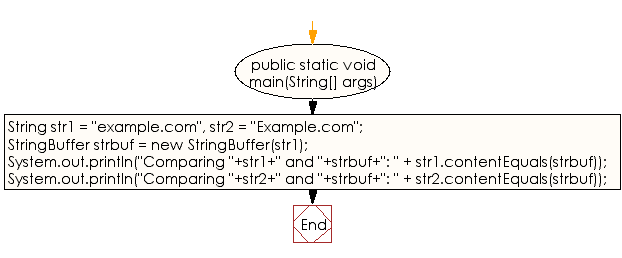
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to compare a given string to the specified character sequence.
Next: Write a Java program to create a new String object with the contents of a character array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics