Java: Divide a string in n equal parts
Java String: Exercise-40 with Solution
Write a Java program to divide a string into n equal parts.
Visual Presentation:
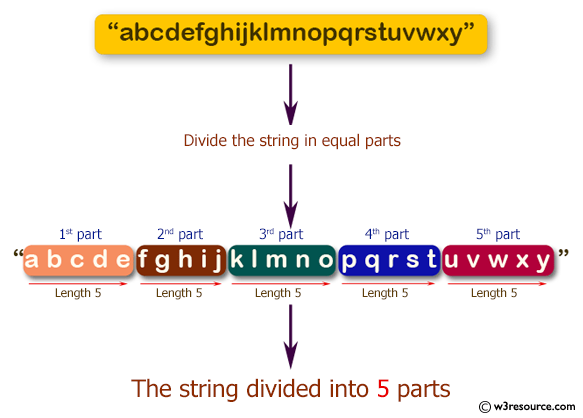
Sample Solution:
Java Code:
// Importing necessary Java utilities.
import java.util.*;
// Define a class named Main.
class Main {
// Method to split the string into 'n' parts.
static void splitString(String str1, int n) {
// Get the length of the string.
int str_size = str1.length();
int part_size;
// Check if the size of the string is divisible by 'n'.
if (str_size % n != 0) {
// If not divisible, print a message and return.
System.out.println("The size of the given string is not divisible by " + n);
return;
} else {
// If divisible, proceed to split the string.
System.out.println("The given string is: " + str1);
System.out.println("The string divided into " + n + " parts and they are: ");
// Calculate the size of each part.
part_size = str_size / n;
// Loop through the string characters.
for (int i = 0; i < str_size; i++) {
// If the current index is a multiple of 'part_size', start a new line.
if (i % part_size == 0)
System.out.println();
// Print the character at the current index.
System.out.print(str1.charAt(i));
}
}
}
// Main method to execute the program.
public static void main(String[] args) {
// Define a string and the number of parts to split.
String str1 = "abcdefghijklmnopqrstuvwxy";
int split_number = 5;
// Call the method to split the string.
splitString(str1, split_number);
}
}
Sample Output:
The given string is: abcdefghijklmnopqrstuvwxy The string divided into 5 parts and they are: abcde fghij klmno pqrst uvwxy
Flowchart:
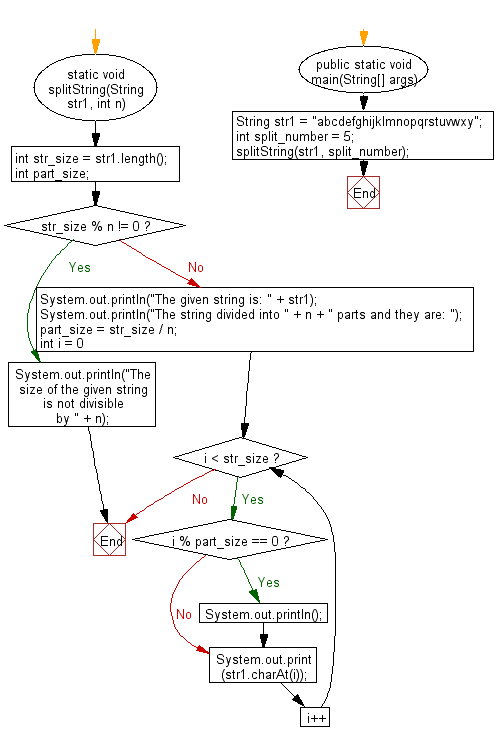
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to find first non repeating character in a string.
Next: Write a Java program to remove duplicate characters from a given string presents in another given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/string/java-string-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics