Java: Repeat a specific number of characters for specific number of times from the last part of a string
Java String: Exercise-78 with Solution
Write a Java program to repeat a specific number of characters for a specific number of times from the last part of a given string.
Visual Presentation:
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to repeat the last 'no_repeat' characters of a string 'stng' 'no_repeat' times
public String lastNchrRepeat(String stng, int no_repeat) {
int l = stng.length(); // Get the length of the given string 'stng'
String new_word = ""; // Initialize an empty string to store the resulting word
// Loop 'no_repeat' times to concatenate the last 'no_repeat' characters of 'stng' 'no_repeat' times
for (int i = 0; i < no_repeat; i++) {
new_word += stng.substring(l - no_repeat, l); // Extract the last 'no_repeat' characters of 'stng' and concatenate them to 'new_word'
}
return new_word; // Return the concatenated string
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "string"; // Given string
int no_char = 3; // Number of times to repeat the last characters
// Display the given string and the number of times to repeat
System.out.println("The given string is: " + str1);
System.out.println("The new string after repetition: " + m.lastNchrRepeat(str1, no_char));
}
}
Sample Output:
The given string is: string The new string after repetition: inginging
Flowchart:
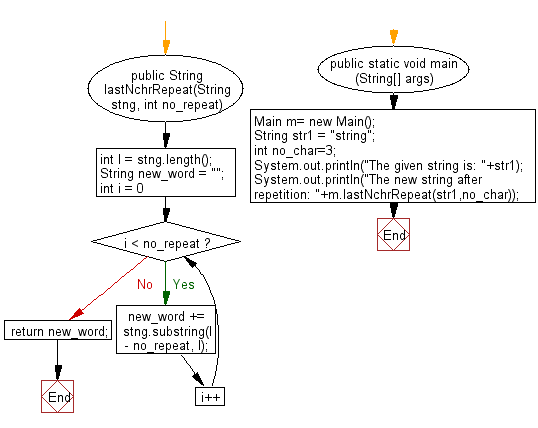
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to add a string with specific number of times separated by a substring.
Next: Write a Java program to create a new string from a given string after removing the 2nd character from the substring of length three starting with 'z' and ending with 'g' presents in the said string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/string/java-string-exercise-78.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics