Java: Check whether the character immediately before and after a specified character is same in a given string
Java String: Exercise-80 with Solution
Write a Java program to check whether the character immediately before and after a specified character is the same in a given string.
Visual Presentation:
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to check if characters before and after '#' are the same
public boolean leftAndRightSame(String stng) {
int l = stng.length(); // Get the length of the given string 'stng'
boolean found = true; // Initialize a boolean variable 'found' as true
// Loop through each character in the given string 'stng'
for (int i = 0; i < l; i++) {
String tmpString = stng.substring(i, i + 1); // Extract each character of 'stng' individually
// Check if the character is '#', and it's not the first or last character
if (tmpString.equals("#") && i > 0 && i < l - 1) {
// Check if the character before '#' is equal to the character after '#'
if (stng.charAt(i - 1) == stng.charAt(i + 1)) {
found = true; // Set 'found' to true if characters before and after '#' are the same
} else {
found = false; // Set 'found' to false if characters before and after '#' are different
}
}
}
return found; // Return the result whether characters before and after '#' are the same or not
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "moon#night"; // Given string
// Display the given string and the result of checking characters before and after '#'
System.out.println("The given string is: " + str1);
System.out.println("The before and after character are same: " + m.leftAndRightSame(str1));
}
}
Sample Output:
The given string is: moon#night The before and after character are same: true The given string is: bat##ball The before and after character are same: false The given string is: #moon#night The before and after character are same: true
Flowchart:
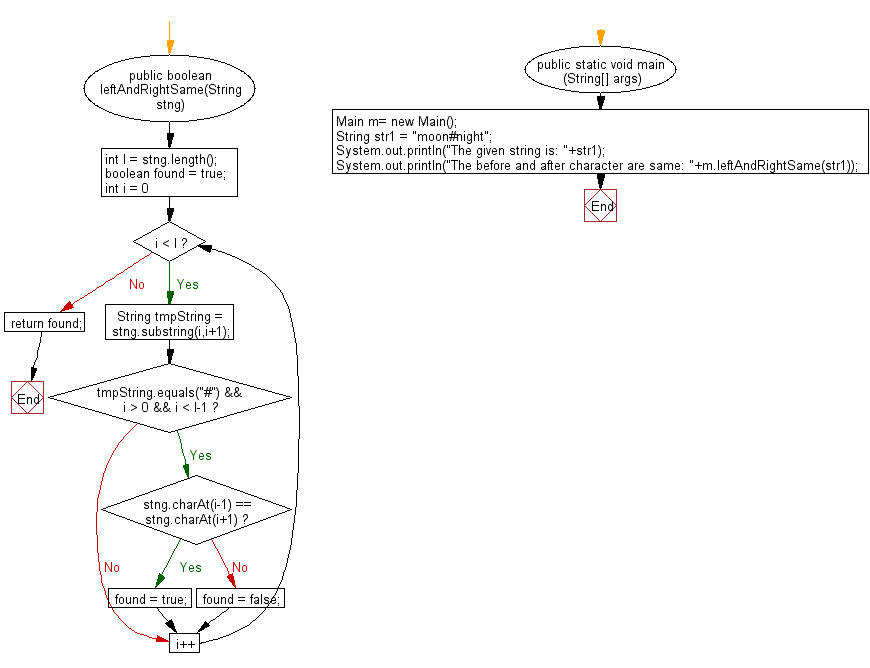
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to create a new string from a given string after removing the 2nd character from the substring of length three starting with 'z' and ending with 'g' presents in the said string.
Next: Write a Java program to check whether two strings of length 3 and 4 appear in same number of times in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics