Java: Make a new string from two given string in such a way that, each character of two string will come respectively
Java String: Exercise-83 with Solution
Write a Java program to create a string from two given strings. This is so that each character of the two strings appears individually in the created string.
Visual Presentation:
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to mix two strings 'stng1' and 'stng2' character-wise
public String stringMixing(String stng1, String stng2) {
int len1 = stng1.length(); // Get the length of the first string 'stng1'
int len2 = stng2.length(); // Get the length of the second string 'stng2'
int max_len = Math.max(len1, len2); // Find the maximum length between two strings
String newstring = ""; // Initialize an empty string to store the mixed string
// Loop through up to the maximum length of the two strings
for (int i = 0; i < max_len; i++) {
// Append the character at index 'i' in 'stng1' if it exists
if (i <= len1 - 1)
newstring += stng1.substring(i, i + 1);
// Append the character at index 'i' in 'stng2' if it exists
if (i <= len2 - 1)
newstring += stng2.substring(i, i + 1);
}
return newstring; // Return the mixed string
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "welcome"; // First string
String str2 = "w3resource"; // Second string
// Display the given strings and the new mixed string
System.out.println("The given strings are: " + str1 + " and " + str2);
System.out.println("The new string is: " + m.stringMixing(str1, str2));
}
}
Sample Output:
The given strings are: welcome and w3resource The new string is: wwe3lrceosmoeurce
Flowchart:
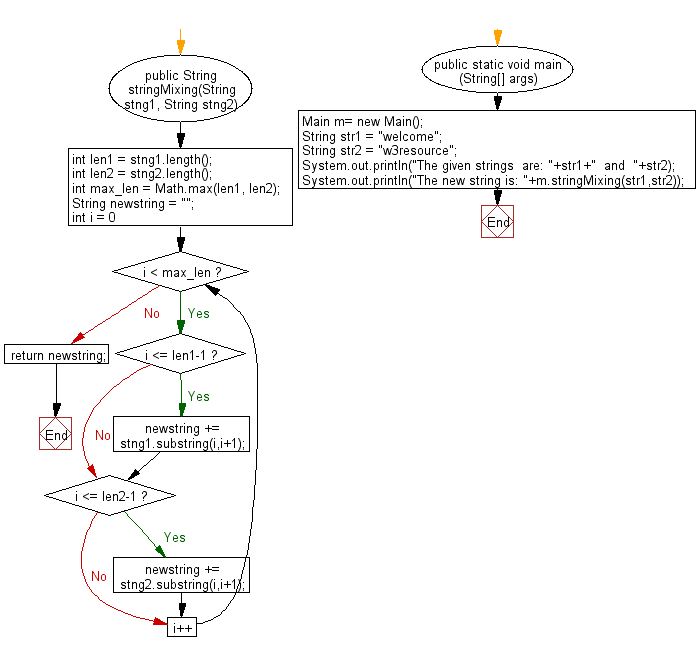
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to create a new string repeating every character twice of a given string.
Next: Write a Java program to make a new string made of p number of characters from the first of a given string and followed by p-1 number characters till the p is greater than zero.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics