Java: Count the number of triples (characters appearing three times in a row) in a given string
Java String: Exercise-86 with Solution
Write a Java program to count the number of triples (characters appearing three times in a row) in a given string.
Sample Solution:
Java Code:
// Define a class named Main
public class Main {
// Method to count the number of triples in the given string
public int noOfTriples(String stng) {
int l = stng.length(); // Get the length of the given string
int ctr = 0; // Initialize a counter for triples
// Loop through the string to check for triples
for (int i = 0; i < l - 2; i++) {
char tmp = stng.charAt(i); // Get the character at index 'i'
// Check if the character at index 'i' is the same as the next two characters
if (tmp == stng.charAt(i + 1) && tmp == stng.charAt(i + 2)) {
ctr++; // Increment the counter if a triple is found
}
}
return ctr; // Return the total count of triples
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "welllcommmmeee"; // Given input string
// Display the given string and the number of triples in it
System.out.println("The given string is: " + str1);
System.out.println("The number of triples in the string is: " + m.noOfTriples(str1));
}
}
Sample Output:
The given string is: welllcommmmeee The number of triples in the string is: 4
Flowchart:
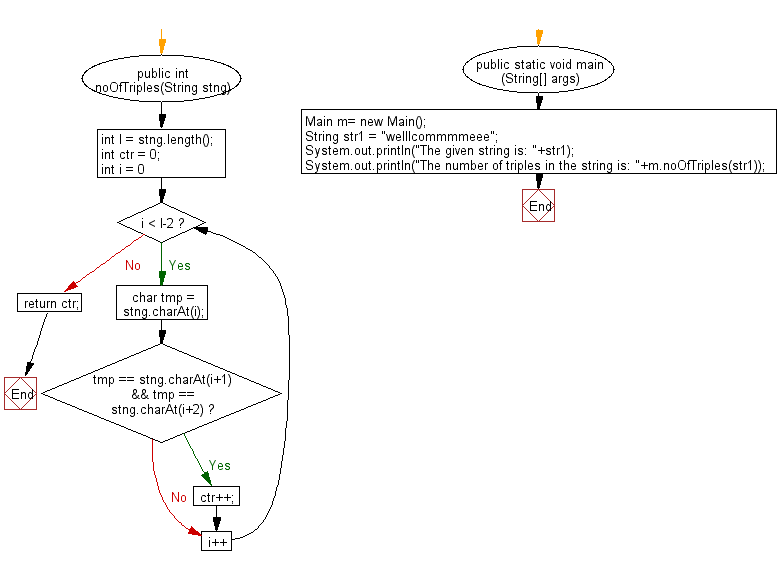
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to make a new string with each character of just before and after of a non-empty substring whichever it appears in a non-empty given string.
Next: Write a Java program to check whether a specified character is happy or not. A character is happy when the same character appears to its left or right in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics