Java: Check whether the first instance of a given character is immediately followed by the same character in a given string
Java String: Exercise-98 with Solution
Write a Java program to check whether the first instance of a given character is immediately followed by the same character in a given string.
Visual Presentation:
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to check if 'z' appears twice in succession in the string
boolean appearTwice(String stng) {
int i = stng.indexOf("z"); // Get the index of the first occurrence of 'z'
if (i == -1) return false; // If 'z' is not found, return false
if (i + 1 >= stng.length()) return false; // If 'z' is at the end of the string, return false
// Check if the character after the first 'z' is also 'z'
return stng.substring(i + 1, i + 2).equals("z");
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "fizzez"; // Given string
// Display the given string and whether 'z' appears twice respectively
System.out.println("The given string is: " + str1);
System.out.println("Is 'z' appear twice respectively? " + m.appearTwice(str1));
}
}
Sample Output:
The given string is: fizzez Is 'z' appear twice respectively? true
Flowchart:
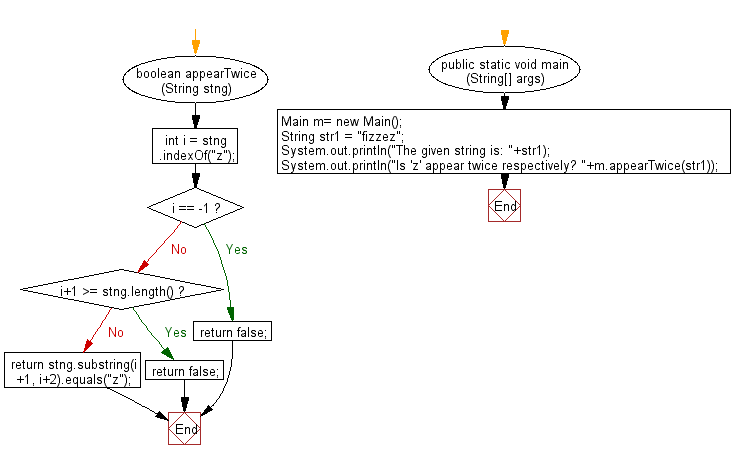
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to return a string with the characters of the index position 0,1,2, 5,6,7, ... from a given string.
Next: Write a Java program to return a new string using every character of even positions from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics