JavaScript Program: Error handling and cleanup with the try-catch-finally statement
JavaScript Error Handling: Exercise-10 with Solution
Try-Catch-Finally Example
Write a JavaScript program that demonstrates the use of the 'try-catch-finally' statement to catch and handle an error, and then execute some cleanup code in the 'finally' block.
Sample Solution:
JavaScript Code:
// Define a function named divide_Numbers that takes two parameters: x and y
function divide_Numbers(x, y) {
// Try block to handle potential errors
try {
// Check if x and y are both numbers
if (typeof x !== 'number' || typeof y !== 'number') {
// If either x or y is not a number, throw a TypeError
throw new TypeError('Invalid arguments. Both arguments should be numbers.');
}
// Check if y is zero
if (y === 0) {
// If y is zero, throw an Error indicating division by zero is not allowed
throw new Error('Invalid divisor. Cannot divide by zero.');
}
// Calculate the result of the division
const result = x / y;
// Log the result to the console
console.log('Result:', result);
} catch (error) {
// If an error occurs during execution, log the error message to the console
console.log('Error:', error.message);
} finally {
// Finally block to execute cleanup code regardless of whether an error occurred or not
console.log('Cleanup code executed.');
}
}
// Example:
// Call the divide_Numbers function with valid arguments
divide_Numbers(10, 2); // Valid division
// Call the divide_Numbers function with a divisor of zero
divide_Numbers(10, 0); // Division by zero
// Call the divide_Numbers function with a non-numeric divisor
divide_Numbers(10, '2'); // Invalid divisor
Output:
"Result:" 5 "Cleanup code executed." "Error:" "Invalid divisor. Cannot divide by zero." "Cleanup code executed." "Error:" "Invalid arguments. Both arguments should be numbers." "Cleanup code executed."
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The function "divide_Numbers()" takes two arguments, x and y. This function performs a division operation and handle potential errors.
- Inside the try block, the code checks if both x and y are numbers using the typeof operator. If either of them is not a number, a TypeError is thrown with the message 'Invalid arguments. Both arguments should be numbers.'.
- Next, check to see if the divisor y is equal to zero. If it is, an Error is thrown with the message 'Invalid divisor. Cannot divide by zero.'.
- If no errors occur, the code performs the division operation x / y and logs the result to the console using console.log('Result:', result).
- If an error is thrown within the try block, execution jumps to the catch block. In the catch block, the error object is caught in the error parameter. Its message is logged to the console using console.log('Error:', error.message).
- Regardless of whether an error occurred or not, the code in the "finally" block is always executed. In this case, the "finally" block contains the statement console.log('Cleanup code executed.'), which logs the message 'Cleanup code executed.' to the console.
Flowchart:
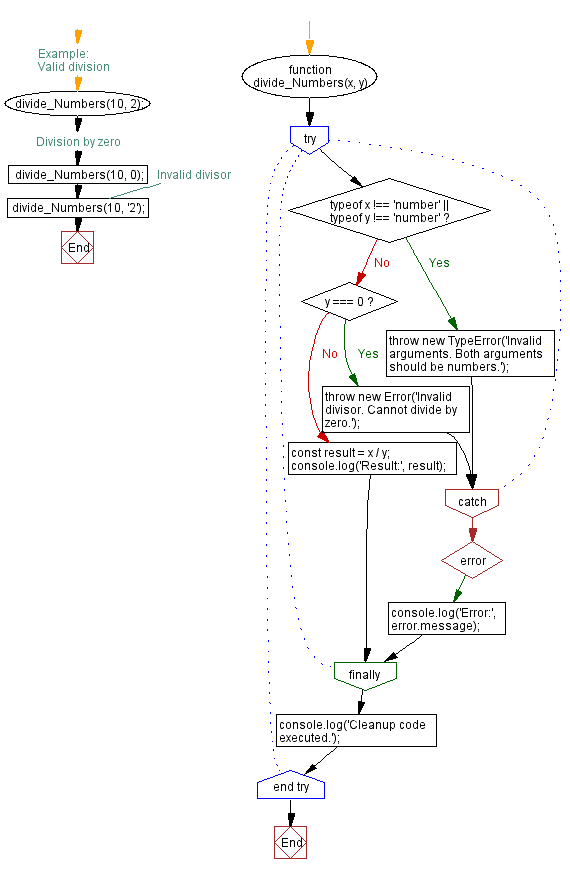
Live Demo:
See the Pen javascript-error-handling-exercise-10 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that simulates resource allocation, triggers an error during processing, and ensures cleanup in the finally block.
- Write a JavaScript function that performs several operations inside a try block, catches any error, and logs a confirmation message in the finally block.
- Write a JavaScript function that contains a nested try-catch-finally structure to simulate multiple layers of error handling with guaranteed cleanup actions.
- Write a JavaScript function that intentionally throws an error to demonstrate the execution order of catch and finally blocks.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Handling URIError with Try-Catch block for invalid URI decoding.
Error Handling Exercises Next: Catching and handling EvalError with the try-catch Block.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.