JavaScript: Test a value, x, against a predicate function
JavaScript fundamental (ES6 Syntax): Exercise-104 with Solution
Conditional Function Application
Write a JavaScript program to test a value, x, against a predicate function. If true, return fn(x). Else, return x.
Return a function expecting a single value, x, that returns the appropriate value based on pred.
- Return a function expecting a single value, x, that returns the appropriate value based on pred.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'when' function to conditionally apply a function to a value.
const when = (pred, whenTrue) => x => (pred(x) ? whenTrue(x) : x);
// Define a function 'doubleEvenNumbers' that doubles even numbers.
const doubleEvenNumbers = when(x => x % 2 === 0, x => x * 2);
// Test the 'doubleEvenNumbers' function with different inputs.
console.log(doubleEvenNumbers(2)); // Output: 4 (2 * 2)
console.log(doubleEvenNumbers(1)); // Output: 1 (unchanged)
Output:
4 1
Visual Presentation:
Flowchart:
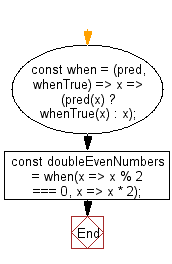
Live Demo:
See the Pen javascript-basic-exercise-104-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that applies a function to a value only if a given predicate returns true, otherwise returns the original value.
- Write a JavaScript function that conditionally transforms a value using a callback based on a provided test function.
- Write a JavaScript program that tests an input value with a predicate and either applies a transformation or leaves it unchanged.
Go to:
PREV : String to Array of Words.
NEXT : Check if Value is a Number.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.