JavaScript: Generate a random hexadecimal color code
JavaScript fundamental (ES6 Syntax): Exercise-11 with Solution
Generate Random Hexadecimal Color Code
Write a JavaScript program to generate a random hexadecimal color code.
- Use Math.random() to generate a random 24-bit (6 * 4bits) hexadecimal number.
- Use bit shifting and then convert it to an hexadecimal string using Number.prototype.toString(16).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `random_hex_color_code` that generates a random hexadecimal color code.
const random_hex_color_code = () => {
// Generate a random number and convert it to hexadecimal string representation.
let n = (Math.random() * 0xfffff * 1000000).toString(16);
// Return the hexadecimal color code with '#' appended.
return '#' + n.slice(0, 6);
};
// Test the function
console.log(random_hex_color_code()); // Output: Random hexadecimal color code
Output:
#2375d4
Flowchart:
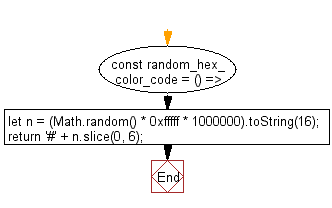
Live Demo:
See the Pen javascript-basic-exercise-1-11 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to extract out the values at the specified indexes from an specified array.
Next: Write a JavaScript program to removes non-printable ASCII characters from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics