JavaScript: Unflatten an object with the paths for keys
JavaScript fundamental (ES6 Syntax): Exercise-112 with Solution
Unflatten Object from Key Paths
Write a JavaScript program to unflatten an object with the paths for keys.
- Use nested Array.prototype.reduce() to convert the flat path to a leaf node.
- Use String.prototype.split('.') to split each key with a dot delimiter and Array.prototype.reduce() to add objects against the keys.
- If the current accumulator already contains a value against a particular key, return its value as the next accumulator.
- Otherwise, add the appropriate key-value pair to the accumulator object and return the value as the accumulator.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'unflattenObject' function.
const unflattenObject = obj =>
Object.keys(obj).reduce((acc, k) => {
// Check if the key contains dot notation.
if (k.indexOf('.') !== -1) {
// Split the key into individual keys based on dot notation.
const keys = k.split('.');
// Use 'JSON.parse' to dynamically construct nested objects based on the keys.
Object.assign(
acc,
JSON.parse(
'{' +
// Create a string representation of nested objects with comma separators.
keys.map((v, i) => (i !== keys.length - 1 ? `"${v}":{` : `"${v}":`)).join('') +
// Add the original value to the innermost object.
obj[k] +
// Close the nested objects with curly braces based on the number of keys.
'}'.repeat(keys.length)
)
);
} else {
// If the key does not contain dot notation, simply assign the value to the object.
acc[k] = obj[k];
}
return acc;
}, {});
// Test the 'unflattenObject' function with a sample object.
console.log(unflattenObject({ 'a.b.c': 1, d: 1 }));
// Output: { a: { b: { c: 1 } }, d: 1 }
Output:
{"a":{"b":{"c":1}},"d":1}
Flowchart:
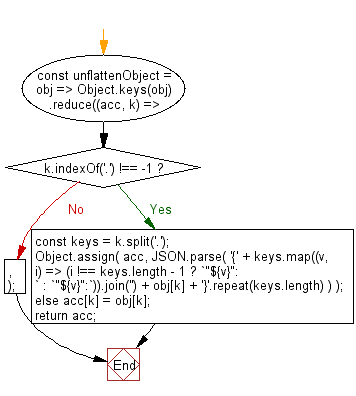
Live Demo:
See the Pen javascript-basic-exercise-112-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a flat object with dotted key paths into a nested object structure.
- Write a JavaScript function that parses key strings containing delimiters and reconstructs the original hierarchical object.
- Write a JavaScript program that transforms an object with compound keys into a multi-level nested object based on the key paths.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to build an array, using an iterator function and an initial seed value.
Next: Write a JavaScript program to unescape escaped HTML characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.