JavaScript: Convert a value to a safe integer
JavaScript fundamental (ES6 Syntax): Exercise-121 with Solution
Convert Value to Safe Integer
Write a JavaScript program to convert a value to a safe integer.
- Use Math.max() and Math.min() to find the closest safe value.
- Use Math.round() to convert to an integer.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'toSafeInteger' function.
const toSafeInteger = num =>
Math.round(Math.max(Math.min(num, Number.MAX_SAFE_INTEGER), Number.MIN_SAFE_INTEGER));
// Test the 'toSafeInteger' function with sample inputs.
console.log(toSafeInteger('3.2')); // Output: 3
console.log(toSafeInteger(Infinity)); // Output: 9007199254740991 (maximum safe integer)
Output:
3 9007199254740991
Visual Presentation:
Flowchart:
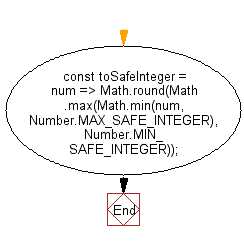
Live Demo:
See the Pen javascript-basic-exercise-121-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts various input values to safe integers, returning a default if conversion fails.
- Write a JavaScript function that validates a value and then coerces it into a safe integer using bitwise operations.
- Write a JavaScript program that checks if a value is within the safe integer range and converts it accordingly.
Go to:
PREV : String to Snake Case.
NEXT : Add Ordinal Suffix to Number.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.