JavaScript: Get removed elements of an given array until the passed function returns true
JavaScript fundamental (ES6 Syntax): Exercise-128 with Solution
Remove Elements Until Function True
Write a JavaScript program to get removed elements of an given array until the passed function returns true.
- Loop through the array, using a for...of loop over Array.prototype.entries() until the returned value from the function is falsy.
- Return the removed elements, using Array.prototype.slice().
- The callback function, fn, accepts a single argument which is the value of the element.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'takeWhile' to retrieve elements from an array until a condition is no longer met
const takeWhile = (arr, func) => {
// Iterate over the array using entries() to get both index and value
for (const [i, val] of arr.entries())
// If the condition function returns false for the current value, return the slice of the array up to this index
if (func(val)) return arr.slice(0, i);
// If the condition is true for all elements, return the original array
return arr;
};
// Test the 'takeWhile' function by retrieving elements from an array while the condition is met
console.log(takeWhile([1, 2, 3, 4], n => n >= 3)); // Output: [1, 2]
Output:
[1,2]
Flowchart:
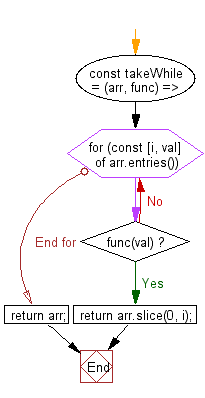
Live Demo:
See the Pen javascript-basic-exercise-128-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes elements from the beginning of an array until a provided function returns true.
- Write a JavaScript function that iteratively shifts elements off an array until the condition is met and returns the removed elements.
- Write a JavaScript program that uses a while loop to drop elements from an array until the predicate evaluates to true for an element.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to iterate over a callback n times.
Next: Write a JavaScript program to get removed elements from the end of an given array until the passed function returns true.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.