JavaScript: Create a shallow clone of an object
JavaScript fundamental (ES6 Syntax): Exercise-146 with Solution
Shallow Clone Object
Write a JavaScript program to create a shallow clone of an object.
- Use Object.assign() and an empty object ({}) to create a shallow clone of the original.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the shallowClone function
const shallowClone = obj => Object.assign({}, obj);
// Create an object 'a'
const a = { x: true, y: 1 };
// Clone object 'a' into object 'b'
const b = shallowClone(a);
// Log the cloned object 'b'
console.log(b); // Output: { x: true, y: 1 }
Output:
{"x":true,"y":1}
Visual Presentation:
Flowchart:
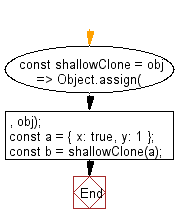
Live Demo:
See the Pen javascript-basic-exercise-146-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to randomize the order of the values of an array, returning a new array.
Next: Write a JavaScript program to serialize a cookie name-value pair into a Set-Cookie header string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-146.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics